Open API¶
An API that is publicly available for anyone to use, allowing external access and utilization of data from a specific platform or service.
What is an API?
API stands for Application Programming Interface.
It is an interface that enables interaction between software systems, providing tools to access specific functionalities or data externally.What is a RestAPI?
An API that adheres to the principles of REST (Representational State Transfer).
It facilitates data exchange between a client and server using the HTTP protocol, typically utilizing JSON, XML, or YAML formats.What is OpenAPI?
A standard specification for designing, documenting, and testing APIs.
It uses standardized formats (JSON or YAML) to describe RESTful API specifications, supporting efficient API development and utilization.
JSON¶
- What is JSON?
JSON stands for JavaScript Object Notation.
It is a lightweight data interchange format commonly used in web environments to transfer data between servers and clients.
One key example of its use is in Rest APIs.
JSON is text-based, easy for humans to read, and simple for machines to parse and generate.
Example of JSON format:{ "id": "01", "language": "Java", "edition": "third", "author": "Herbert Schildt" }
GOOGLE_API_KEY = 'YOUR KEY'
GOOGLE_CX = 'YOUR CX'
import requests
# Construct the Google Custom Search API URL with the given API key, search engine ID, and query parameters
google_open_api = f'https://www.googleapis.com/customsearch/v1?key={GOOGLE_API_KEY}&cx={GOOGLE_CX}&q=IPhone'
# Send a GET request to the Google Custom Search API
res = requests.get(google_open_api)
# Check if the API request was successful (HTTP status code 200)
if res.status_code == 200:
# Parse the response JSON data
data = res.json()
# Iterate through the search result items and print their index, title, and link
for index, item in enumerate(data['items']):
print(f"{index + 1}, {item['title']}, {item['link']}")
else:
# Print the error code if the request was unsuccessful
print("Error Code:", res.status_code)
1, iPhone - Apple, https://www.apple.com/iphone/ 2, iPhone - Wikipedia, https://en.wikipedia.org/wiki/IPhone 3, Apple, https://www.apple.com/ 4, Update your iPhone or iPad - Apple Support, https://support.apple.com/en-us/118575 5, iPhone - Compare Models - Apple, https://www.apple.com/iphone/compare/ 6, Apple iPhone - Walmart.com, https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173 7, r/iPhone, https://www.reddit.com/r/iphone/ 8, Telegram Messenger on the App Store, https://apps.apple.com/us/app/telegram-messenger/id686449807 9, Use parental controls on your child's iPhone or iPad - Apple Support, https://support.apple.com/en-us/105121 10, WhatsApp Messenger on the App Store, https://apps.apple.com/us/app/whatsapp-messenger/id310633997
search_result = """
{
"kind": "customsearch#search",
"url": {
"type": "application/json",
"template": "https://www.googleapis.com/customsearch/v1?q={searchTerms}&num={count?}&start={startIndex?}&lr={language?}&safe={safe?}&cx={cx?}&sort={sort?}&filter={filter?}&gl={gl?}&cr={cr?}&googlehost={googleHost?}&c2coff={disableCnTwTranslation?}&hq={hq?}&hl={hl?}&siteSearch={siteSearch?}&siteSearchFilter={siteSearchFilter?}&exactTerms={exactTerms?}&excludeTerms={excludeTerms?}&linkSite={linkSite?}&orTerms={orTerms?}&dateRestrict={dateRestrict?}&lowRange={lowRange?}&highRange={highRange?}&searchType={searchType}&fileType={fileType?}&rights={rights?}&imgSize={imgSize?}&imgType={imgType?}&imgColorType={imgColorType?}&imgDominantColor={imgDominantColor?}&alt=json"
},
"queries": {
"request": [
{
"title": "Google Custom Search - Iphone",
"totalResults": "6290000000",
"searchTerms": "Iphone",
"count": 10,
"startIndex": 1,
"inputEncoding": "utf8",
"outputEncoding": "utf8",
"safe": "off",
"cx": "1592a8f069c744b73"
}
],
"nextPage": [
{
"title": "Google Custom Search - Iphone",
"totalResults": "6290000000",
"searchTerms": "Iphone",
"count": 10,
"startIndex": 11,
"inputEncoding": "utf8",
"outputEncoding": "utf8",
"safe": "off",
"cx": "1592a8f069c744b73"
}
]
},
"context": {
"title": "ppp"
},
"searchInformation": {
"searchTime": 0.237241,
"formattedSearchTime": "0.24",
"totalResults": "6290000000",
"formattedTotalResults": "6,290,000,000"
},
"items": [
{
"kind": "customsearch#result",
"title": "iPhone - Apple",
"htmlTitle": "<b>iPhone</b> - Apple",
"link": "https://www.apple.com/iphone/",
"displayLink": "www.apple.com",
"snippet": "Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.",
"htmlSnippet": "Designed for Apple Intelligence. Discover the new <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, and <b>iPhone</b> 16 Plus.",
"formattedUrl": "https://www.apple.com/iphone/",
"htmlFormattedUrl": "https://www.apple.com/<b>iphone</b>/",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSTP05LrdG82MHIYno9vsxK6v__4F7GDtXjDnOCzLzKXdMSrHVkT5J4BNA&s",
"width": "310",
"height": "163"
}
],
"BreadcrumbList": [
{}
],
"metatags": [
{
"analytics-s-bucket-1": "applestoreww",
"og:image": "https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100",
"analytics-s-bucket-0": "applestoreww",
"og:type": "website",
"twitter:card": "summary_large_image",
"og:site_name": "Apple",
"al:ios:app_name": "Apple Store",
"globalnav-store-key": "SFX9YPYY9PPXCU9KH",
"og:title": "iPhone",
"al:ios:url": "https://www.apple.com/us/xc/iphone?cid=AOS_ASA",
"og:description": "Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.",
"al:ios:app_store_id": "375380948",
"analytics-s-channel": "iphone",
"twitter:site": "@Apple",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"ac:pricing-alias": "iphone-16-pro=IPHONE16PRO",
"og:locale": "en_US",
"ac:tradein-alias": "tradein-iphone12=slug:model_iphone_12",
"position": "1",
"og:url": "https://www.apple.com/iphone/",
"analytics-track": "iphone - index/tab",
"analytics-s-bucket-2": "applestoreww"
}
],
"cse_image": [
{
"src": "https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100"
}
]
}
},
{
"kind": "customsearch#result",
"title": "iPhone - Wikipedia",
"htmlTitle": "<b>iPhone</b> - Wikipedia",
"link": "https://en.wikipedia.org/wiki/IPhone",
"displayLink": "en.wikipedia.org",
"snippet": "iPhone ... For other uses, see iPhone (disambiguation). The iPhone is a line of smartphones developed and marketed by Apple that run iOS, the company's own mobile ...",
"htmlSnippet": "<b>iPhone</b> ... For other uses, see <b>iPhone</b> (disambiguation). The <b>iPhone</b> is a line of smartphones developed and marketed by Apple that run iOS, the company's own mobile ...",
"formattedUrl": "https://en.wikipedia.org/wiki/IPhone",
"htmlFormattedUrl": "https://en.wikipedia.org/wiki/<b>IPhone</b>",
"pagemap": {
"metatags": [
{
"referrer": "origin",
"og:image": "https://upload.wikimedia.org/wikipedia/en/e/ee/Front_%26_Back_Face_of_iPhone_16_Pro_Max.png",
"theme-color": "#eaecf0",
"og:image:width": "1200",
"og:type": "website",
"viewport": "width=device-width, initial-scale=1.0, user-scalable=yes, minimum-scale=0.25, maximum-scale=5.0",
"og:title": "iPhone - Wikipedia",
"og:image:height": "1423",
"format-detection": "telephone=no"
}
],
"hproduct": [
{
"fn": "iPhone",
"url": "apple.com/iphone"
}
]
}
},
{
"kind": "customsearch#result",
"title": "Apple",
"htmlTitle": "Apple",
"link": "https://www.apple.com/",
"displayLink": "www.apple.com",
"snippet": "Discover the innovative world of Apple and shop everything iPhone, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, ...",
"htmlSnippet": "Discover the innovative world of Apple and shop everything <b>iPhone</b>, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, ...",
"formattedUrl": "https://www.apple.com/",
"htmlFormattedUrl": "https://www.apple.com/",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRU17BvguBEtrzqLvgQkLdknkk8vZsRlqab1b8SC5XiOzqGTdFtArLLMw&s=0",
"width": "150",
"height": "79"
}
],
"metatags": [
{
"analytics-s-bucket-1": "applestoreww",
"analytics-s-bucket-0": "applestoreww",
"og:image": "https://www.apple.com/ac/structured-data/images/open_graph_logo.png?202110180743",
"og:type": "website",
"og:site_name": "Apple",
"globalnav-store-key": "SFX9YPYY9PPXCU9KH",
"og:title": "Apple",
"og:description": "Discover the innovative world of Apple and shop everything iPhone, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, and expert device support.",
"analytics-s-channel": "homepage",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"og:locale": "en_US",
"og:url": "https://www.apple.com/",
"analytics-track": "Apple - Index/Tab",
"analytics-s-bucket-2": "applestoreww"
}
],
"cse_image": [
{
"src": "https://www.apple.com/ac/structured-data/images/open_graph_logo.png?202110180743"
}
]
}
},
{
"kind": "customsearch#result",
"title": "Apple iPhone - Walmart.com",
"htmlTitle": "Apple <b>iPhone</b> - Walmart.com",
"link": "https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173",
"displayLink": "www.walmart.com",
"snippet": "Shop for Apple iPhone at Walmart and save.",
"htmlSnippet": "Shop for Apple <b>iPhone</b> at Walmart and save.",
"formattedUrl": "https://www.walmart.com/browse/cell...iphone/1105910_7551331_112717...",
"htmlFormattedUrl": "https://www.walmart.com/browse/cell...<b>iphone</b>/1105910_7551331_112717...",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRIpWNIuWqABoeCNoSiltxtZvq3G-HeNHvTzQGF05buRP2PsFt9_9FJ_hU&s",
"width": "180",
"height": "180"
}
],
"metatags": [
{
"og:image": "https://i5.walmartimages.com/seo/Total-by-Verizon-Apple-iPhone-12-64GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon_66b2853b-6cb5-4f20-b73a-b60b39b6de44.6b3bf83a920058a47342318925f1dc2b.jpeg?odnHeight=180&odnWidth=180&odnBg=FFFFFF",
"next-head-count": "27",
"fb:app_id": "105223049547814",
"og:type": "Website",
"og:site_name": "Walmart.com",
"viewport": "width=device-width, initial-scale=1.0, minimum-scale=1, interactive-widget=resizes-content",
"og:title": "Apple iPhone - Walmart.com",
"og:url": "https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173",
"og:description": "Shop for Apple iPhone at Walmart and save."
}
],
"cse_image": [
{
"src": "https://i5.walmartimages.com/seo/Total-by-Verizon-Apple-iPhone-12-64GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon_66b2853b-6cb5-4f20-b73a-b60b39b6de44.6b3bf83a920058a47342318925f1dc2b.jpeg?odnHeight=180&odnWidth=180&odnBg=FFFFFF"
}
],
"hproduct": [
{
"fn": "Your Privacy Choices",
"photo": "https://i5.walmartimages.com/dfwrs/76316474-d730/k2-_3c5ba298-4f19-46be-9fc3-ac49225d19bd.v1.png"
}
]
}
},
{
"kind": "customsearch#result",
"title": "iPhone - Compare Models - Apple",
"htmlTitle": "<b>iPhone</b> - Compare Models - Apple",
"link": "https://www.apple.com/iphone/compare/",
"displayLink": "www.apple.com",
"snippet": "Compare features and technical specifications for iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, iPhone 16 Plus, iPhone SE, and many more.",
"htmlSnippet": "Compare features and technical specifications for <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, <b>iPhone</b> 16 Plus, <b>iPhone</b> SE, and many more.",
"formattedUrl": "https://www.apple.com/iphone/compare/",
"htmlFormattedUrl": "https://www.apple.com/<b>iphone</b>/compare/",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTzLb5dy3cvHFpnrcOVU29mrKsUkOUb4QmCFXw_T_mwVA29b8M2nHk0tUQ&s",
"width": "310",
"height": "163"
}
],
"BreadcrumbList": [
{}
],
"metatags": [
{
"analytics-s-bucket-1": "applestoreww",
"og:image": "https://www.apple.com/v/iphone/compare/ah/images/meta/compare__b5xf30djrsia_og.png?202412111328",
"analytics-s-bucket-0": "applestoreww",
"og:type": "website",
"og:site_name": "Apple",
"globalnav-store-key": "SFX9YPYY9PPXCU9KH",
"og:title": "iPhone - Compare Models",
"og:description": "Compare features and technical specifications for iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, iPhone 16 Plus, iPhone SE, and many more.",
"analytics-s-channel": "iphone.tab+other",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"ac-custom-local-desc-start": "Compare features and technical specifications for the",
"og:locale": "en_US",
"ac:tradein-alias": "tradein-iphone12=slug:model_iphone_12",
"position": "1",
"og:url": "https://www.apple.com/iphone/compare/",
"ac-custom-local-desc-end": "and many more.",
"analytics-track": "iphone - compare",
"analytics-s-bucket-2": "applestoreww"
}
],
"cse_image": [
{
"src": "https://www.apple.com/v/iphone/compare/ah/images/meta/compare__b5xf30djrsia_og.png?202412111328"
}
]
}
},
{
"kind": "customsearch#result",
"title": "Update your iPhone or iPad - Apple Support",
"htmlTitle": "Update your <b>iPhone</b> or iPad - Apple Support",
"link": "https://support.apple.com/en-us/118575",
"displayLink": "support.apple.com",
"snippet": "Sep 19, 2024 ... Update your iPhone or iPad wirelessly · Back up your device using iCloud or your computer. · Plug your device into power and connect to the ...",
"htmlSnippet": "Sep 19, 2024 <b>...</b> Update your <b>iPhone</b> or iPad wirelessly · Back up your device using iCloud or your computer. · Plug your device into power and connect to the ...",
"formattedUrl": "https://support.apple.com/en-us/118575",
"htmlFormattedUrl": "https://support.apple.com/en-us/118575",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSafPk1caLY6LV4JEVDvRjg3kmVQamcLheqsPNRq1T3XBDfAjjYR78xl3E&s",
"width": "310",
"height": "163"
}
],
"Organization": [
{
"name": "Apple",
"telephone": "1-800-692-7753"
}
],
"BreadcrumbList": [
{}
],
"metatags": [
{
"og:image": "https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg",
"og:image:width": "630",
"twitter:card": "summary_large_image",
"globalnav-search-field[locale]": "en_US",
"og:site_name": "Apple Support",
"globalnav-store-key": "S2A49YFKJF2JAT22K",
"globalnav-search-field[src]": "globalnav_support",
"og:description": "Learn how to update your iPhone or iPad to the latest version of iOS or iPadOS.",
"twitter:image": "https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg",
"globalnav-search-field[placeholder]": "Search Support",
"twitter:site": "@AppleSupport",
"globalnav-search-field[type]": "organic",
"globalnav-search-field[page]": "search",
"og:type": "article",
"twitter:title": "Update your iPhone or iPad",
"og:title": "Update your iPhone or iPad - Apple Support",
"og:image:height": "1200",
"globalnav-search-field[name]": "q",
"telephone": "1-800-692-7753",
"globalnav-search-field[action]": "https://support.apple.com/kb/index",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"twitter:description": "Learn how to update your iPhone or iPad to the latest version of iOS or iPadOS.",
"og:locale": "en_US",
"name": "Apple",
"position": "1",
"og:url": "https://support.apple.com/en-us/118575"
}
],
"cse_image": [
{
"src": "https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg"
}
]
}
},
{
"kind": "customsearch#result",
"title": "Telegram Messenger on the App Store",
"htmlTitle": "Telegram Messenger on the App Store",
"link": "https://apps.apple.com/us/app/telegram-messenger/id686449807",
"displayLink": "apps.apple.com",
"snippet": "iPhone: Requires iOS 12.0 or later. iPad: Requires iPadOS 12.0 or later. iPod touch: Requires iOS 12.0 or later. Apple Vision: Requires visionOS 1.0 or later ...",
"htmlSnippet": "<b>iPhone</b>: Requires iOS 12.0 or later. iPad: Requires iPadOS 12.0 or later. iPod touch: Requires iOS 12.0 or later. Apple Vision: Requires visionOS 1.0 or later ...",
"formattedUrl": "https://apps.apple.com/us/app/telegram-messenger/id686449807",
"htmlFormattedUrl": "https://apps.apple.com/us/app/telegram-messenger/id686449807",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTi86mx0K6hvU0r_kJDP7X1_tuMFhG6srkwXefJd519GTppZyyYuWQrKI4&s",
"width": "310",
"height": "163"
}
],
"metatags": [
{
"og:image": "https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png",
"og:image:width": "1200",
"twitter:card": "summary_large_image",
"og:site_name": "App Store",
"applicable-device": "pc,mobile",
"og:image:type": "image/png",
"og:description": "Pure instant messaging — simple, fast, secure, and synced across all your devices. One of the top 5 most downloaded apps in the world with over 950 million active users.\n\nFAST: Telegram is the fastest messaging app on the market, connecting people via a unique, distributed network of data centers ar…",
"og:image:secure_url": "https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png",
"twitter:image": "https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x600wa.png",
"web-experience-app/config/environment": "%7B%22appVersion%22%3A1%2C%22modulePrefix%22%3A%22web-experience-app%22%2C%22environment%22%3A%22production%22%2C%22rootURL%22%3A%22%2F%22%2C%22locationType%22%3A%22history-hash-router-scroll%22%2C%22historySupportMiddleware%22%3Atrue%2C%22EmberENV%22%3A%7B%22FEATURES%22%3A%7B%7D%2C%22EXTEND_PROTOTYPES%22%3A%7B%22Date%22%3Afalse%7D%2C%22_APPLICATION_TEMPLATE_WRAPPER%22%3Afalse%2C%22_DEFAULT_ASYNC_OBSERVERS%22%3Atrue%2C%22_JQUERY_INTEGRATION%22%3Afalse%2C%22_TEMPLATE_ONLY_GLIMMER_COMPONENTS%22%3Atrue%7D%2C%22APP%22%3A%7B%22PROGRESS_BAR_DELAY%22%3A3000%2C%22CLOCK_INTERVAL%22%3A1000%2C%22LOADING_SPINNER_SPY%22%3Atrue%2C%22BREAKPOINTS%22%3A%7B%22large%22%3A%7B%22min%22%3A1069%2C%22content%22%3A980%7D%2C%22medium%22%3A%7B%22min%22%3A735%2C%22max%22%3A1068%2C%22content%22%3A692%7D%2C%22small%22%3A%7B%22min%22%3A320%2C%22max%22%3A734%2C%22content%22%3A280%7D%7D%2C%22buildVariant%22%3A%22apps%22%2C%22name%22%3A%22web-experience-app%22%2C%22version%22%3A%222450.1.0%2B6799f125%22%7D%2C%22MEDIA_API%22%3A%7B%22token%22%3",
"twitter:image:alt": "Telegram Messenger on the App Store",
"twitter:site": "@AppStore",
"og:image:alt": "Telegram Messenger on the App Store",
"og:type": "website",
"twitter:title": "Telegram Messenger",
"og:title": "Telegram Messenger",
"og:image:height": "630",
"version": "2450.1.0",
"globalnav-search-suggestions-enabled": "false",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"ac-gn-search-suggestions-enabled": "false",
"twitter:description": "Pure instant messaging — simple, fast, secure, and synced across all your devices. One of the top 5 most downloaded apps in the world with over 950 million active users.\n\nFAST: Telegram is the fastest messaging app on the market, connecting people via a unique, distributed network of data centers ar…",
"og:locale": "en_US",
"apple:content_id": "686449807",
"og:url": "https://apps.apple.com/us/app/telegram-messenger/id686449807"
}
],
"cse_image": [
{
"src": "https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png"
}
]
}
},
{
"kind": "customsearch#result",
"title": "r/iPhone",
"htmlTitle": "r/<b>iPhone</b>",
"link": "https://www.reddit.com/r/iphone/",
"displayLink": "www.reddit.com",
"snippet": "r/iphone: Reddit's little corner for iPhone lovers (and some people who just mildly enjoy it…)",
"htmlSnippet": "r/<b>iphone</b>: Reddit's little corner for <b>iPhone</b> lovers (and some people who just mildly enjoy it…)",
"formattedUrl": "https://www.reddit.com/r/iphone/",
"htmlFormattedUrl": "https://www.reddit.com/r/<b>iphone</b>/",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSIdavNM8sk5fHPLXO2OdOBUBDBfHfnuGH6tXC7xcmo_xj1hy-QzHPqdBkx&s",
"width": "227",
"height": "222"
}
],
"metatags": [
{
"og:image": "https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa",
"theme-color": "#000000",
"og:image:width": "256",
"og:type": "website",
"twitter:card": "summary",
"twitter:title": "r/iphone",
"og:site_name": "Reddit",
"og:title": "r/iphone",
"og:image:height": "256",
"bingbot": "noarchive",
"msapplication-navbutton-color": "#000000",
"og:description": "Reddit’s little corner for iPhone lovers (and some people who just mildly enjoy it…)",
"twitter:image": "https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa",
"apple-mobile-web-app-status-bar-style": "black",
"twitter:site": "@reddit",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"apple-mobile-web-app-capable": "yes",
"og:ttl": "600",
"og:url": "https://www.reddit.com/r/iphone/"
}
],
"cse_image": [
{
"src": "https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa"
}
]
}
},
{
"kind": "customsearch#result",
"title": "iPhone Repair and Service - Apple Support",
"htmlTitle": "<b>iPhone</b> Repair and Service - Apple Support",
"link": "https://support.apple.com/iphone/repair",
"displayLink": "support.apple.com",
"snippet": "Use our “Get an Estimate” tool to review potential costs if you get service directly from Apple. If you go to another service provider, they can set their own ...",
"htmlSnippet": "Use our “Get an Estimate” tool to review potential costs if you get service directly from Apple. If you go to another service provider, they can set their own ...",
"formattedUrl": "https://support.apple.com/iphone/repair",
"htmlFormattedUrl": "https://support.apple.com/<b>iphone</b>/repair",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTdEyIxqj7ExppNkJKjZtJuqaGqgVBPzm3zYd6LUNtH7u-uJZtEqxfk3Mar&s",
"width": "263",
"height": "192"
}
],
"Organization": [
{
"name": "Apple",
"telephone": "1-800-692-7753"
}
],
"BreadcrumbList": [
{}
],
"metatags": [
{
"og:type": "article",
"globalnav-search-field[locale]": "en_US",
"og:site_name": "Apple Support",
"globalnav-store-key": "S2A49YFKJF2JAT22K",
"og:title": "iPhone Repair and Service - Apple Support",
"globalnav-search-field[src]": "globalnav_support",
"globalnav-search-field[name]": "q",
"telephone": "1-800-692-7753",
"og:description": "Need to repair your iPhone? See your service options, their costs by coverage type, and how long they take.",
"globalnav-search-field[placeholder]": "Search Support",
"globalnav-search-field[action]": "https://support.apple.com/kb/index",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"og:locale": "en_US",
"globalnav-search-field[type]": "organic",
"name": "Apple",
"globalnav-search-field[page]": "search",
"position": "1",
"og:url": "https://support.apple.com/iphone/repair"
}
],
"cse_image": [
{
"src": "https://cdsassets.apple.com/live/7WUAS350/sfaq/SFAQ-header-iphone_2x-gray.png"
}
]
}
},
{
"kind": "customsearch#result",
"title": "WhatsApp Messenger on the App Store",
"htmlTitle": "WhatsApp Messenger on the App Store",
"link": "https://apps.apple.com/us/app/whatsapp-messenger/id310633997",
"displayLink": "apps.apple.com",
"snippet": "Dec 16, 2024 ... This app is available only on the App Store for iPhone, iPad, and Mac. WhatsApp Messenger 12+. Simple. Reliable. Private. WhatsApp Inc. #2 in ...",
"htmlSnippet": "Dec 16, 2024 <b>...</b> This app is available only on the App Store for <b>iPhone</b>, iPad, and Mac. WhatsApp Messenger 12+. Simple. Reliable. Private. WhatsApp Inc. #2 in ...",
"formattedUrl": "https://apps.apple.com/us/app/whatsapp-messenger/id310633997",
"htmlFormattedUrl": "https://apps.apple.com/us/app/whatsapp-messenger/id310633997",
"pagemap": {
"cse_thumbnail": [
{
"src": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSgvcpvLlQU9FI1s23H-2B5gelrpNToJqc1vHO_tdCb7vfiagpC5c5E3BM&s",
"width": "310",
"height": "163"
}
],
"metatags": [
{
"og:image": "https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png",
"og:image:width": "1200",
"twitter:card": "summary_large_image",
"og:site_name": "App Store",
"applicable-device": "pc,mobile",
"og:image:type": "image/png",
"og:description": "With WhatsApp for Mac, you can conveniently sync all your chats to your computer. Message privately, make calls and share files with your friends, family and colleagues.",
"og:image:secure_url": "https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png",
"twitter:image": "https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x600wa.png",
"web-experience-app/config/environment": "%7B%22appVersion%22%3A1%2C%22modulePrefix%22%3A%22web-experience-app%22%2C%22environment%22%3A%22production%22%2C%22rootURL%22%3A%22%2F%22%2C%22locationType%22%3A%22history-hash-router-scroll%22%2C%22historySupportMiddleware%22%3Atrue%2C%22EmberENV%22%3A%7B%22FEATURES%22%3A%7B%7D%2C%22EXTEND_PROTOTYPES%22%3A%7B%22Date%22%3Afalse%7D%2C%22_APPLICATION_TEMPLATE_WRAPPER%22%3Afalse%2C%22_DEFAULT_ASYNC_OBSERVERS%22%3Atrue%2C%22_JQUERY_INTEGRATION%22%3Afalse%2C%22_TEMPLATE_ONLY_GLIMMER_COMPONENTS%22%3Atrue%7D%2C%22APP%22%3A%7B%22PROGRESS_BAR_DELAY%22%3A3000%2C%22CLOCK_INTERVAL%22%3A1000%2C%22LOADING_SPINNER_SPY%22%3Atrue%2C%22BREAKPOINTS%22%3A%7B%22large%22%3A%7B%22min%22%3A1069%2C%22content%22%3A980%7D%2C%22medium%22%3A%7B%22min%22%3A735%2C%22max%22%3A1068%2C%22content%22%3A692%7D%2C%22small%22%3A%7B%22min%22%3A320%2C%22max%22%3A734%2C%22content%22%3A280%7D%7D%2C%22buildVariant%22%3A%22apps%22%2C%22name%22%3A%22web-experience-app%22%2C%22version%22%3A%222450.1.0%2B6799f125%22%7D%2C%22MEDIA_API%22%3A%7B%22token%22%3",
"twitter:image:alt": "WhatsApp Messenger on the App Store",
"twitter:site": "@AppStore",
"og:image:alt": "WhatsApp Messenger on the App Store",
"og:type": "website",
"twitter:title": "WhatsApp Messenger",
"og:title": "WhatsApp Messenger",
"og:image:height": "630",
"version": "2450.1.0",
"globalnav-search-suggestions-enabled": "false",
"viewport": "width=device-width, initial-scale=1, viewport-fit=cover",
"ac-gn-search-suggestions-enabled": "false",
"twitter:description": "With WhatsApp for Mac, you can conveniently sync all your chats to your computer. Message privately, make calls and share files with your friends, family and colleagues.",
"og:locale": "en_US",
"apple:content_id": "310633997",
"og:url": "https://apps.apple.com/us/app/whatsapp-messenger/id310633997"
}
],
"cse_image": [
{
"src": "https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png"
}
]
}
}
]
}
"""
import json
# Try to parse the 'search_result' JSON string and access its contents
try:
# Parse JSON data from the string 'search_result'
json_data = json.loads(search_result, strict=False)
# Print the parsed JSON data for debugging or inspection
print(f"JSON data: {json_data}")
# Extract and print the 'lastBuildDate' key from the JSON object
print(f"LastBuildDate: {json_data.get('lastBuildDate')}")
# Extract and print the 'items' key from the JSON object
print(f"Items: {json_data.get('items')}")
# Access and print the first item in the 'items' list
print(f"First Item: {json_data['items'][0]}")
# Extract and print the 'title' of the first item in the 'items' list
print(f"title of First Item: {json_data['items'][0]['title']}")
# Extract and print the 'link' of the first item in the 'items' list
print(f"Link of First Item: {json_data['items'][0]['link']}")
# Handle JSON decoding errors if the string is not valid JSON
except json.JSONDecodeError as e:
# Print an error message indicating JSON decoding failure
print(f"Error decoding JSON: {e}")
JSON data: {'kind': 'customsearch#search', 'url': {'type': 'application/json', 'template': 'https://www.googleapis.com/customsearch/v1?q={searchTerms}&num={count?}&start={startIndex?}&lr={language?}&safe={safe?}&cx={cx?}&sort={sort?}&filter={filter?}&gl={gl?}&cr={cr?}&googlehost={googleHost?}&c2coff={disableCnTwTranslation?}&hq={hq?}&hl={hl?}&siteSearch={siteSearch?}&siteSearchFilter={siteSearchFilter?}&exactTerms={exactTerms?}&excludeTerms={excludeTerms?}&linkSite={linkSite?}&orTerms={orTerms?}&dateRestrict={dateRestrict?}&lowRange={lowRange?}&highRange={highRange?}&searchType={searchType}&fileType={fileType?}&rights={rights?}&imgSize={imgSize?}&imgType={imgType?}&imgColorType={imgColorType?}&imgDominantColor={imgDominantColor?}&alt=json'}, 'queries': {'request': [{'title': 'Google Custom Search - Iphone', 'totalResults': '6290000000', 'searchTerms': 'Iphone', 'count': 10, 'startIndex': 1, 'inputEncoding': 'utf8', 'outputEncoding': 'utf8', 'safe': 'off', 'cx': '1592a8f069c744b73'}], 'nextPage': [{'title': 'Google Custom Search - Iphone', 'totalResults': '6290000000', 'searchTerms': 'Iphone', 'count': 10, 'startIndex': 11, 'inputEncoding': 'utf8', 'outputEncoding': 'utf8', 'safe': 'off', 'cx': '1592a8f069c744b73'}]}, 'context': {'title': 'ppp'}, 'searchInformation': {'searchTime': 0.237241, 'formattedSearchTime': '0.24', 'totalResults': '6290000000', 'formattedTotalResults': '6,290,000,000'}, 'items': [{'kind': 'customsearch#result', 'title': 'iPhone - Apple', 'htmlTitle': '<b>iPhone</b> - Apple', 'link': 'https://www.apple.com/iphone/', 'displayLink': 'www.apple.com', 'snippet': 'Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.', 'htmlSnippet': 'Designed for Apple Intelligence. Discover the new <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, and <b>iPhone</b> 16 Plus.', 'formattedUrl': 'https://www.apple.com/iphone/', 'htmlFormattedUrl': 'https://www.apple.com/<b>iphone</b>/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSTP05LrdG82MHIYno9vsxK6v__4F7GDtXjDnOCzLzKXdMSrHVkT5J4BNA&s', 'width': '310', 'height': '163'}], 'BreadcrumbList': [{}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'og:image': 'https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100', 'analytics-s-bucket-0': 'applestoreww', 'og:type': 'website', 'twitter:card': 'summary_large_image', 'og:site_name': 'Apple', 'al:ios:app_name': 'Apple Store', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'iPhone', 'al:ios:url': 'https://www.apple.com/us/xc/iphone?cid=AOS_ASA', 'og:description': 'Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.', 'al:ios:app_store_id': '375380948', 'analytics-s-channel': 'iphone', 'twitter:site': '@Apple', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac:pricing-alias': 'iphone-16-pro=IPHONE16PRO', 'og:locale': 'en_US', 'ac:tradein-alias': 'tradein-iphone12=slug:model_iphone_12', 'position': '1', 'og:url': 'https://www.apple.com/iphone/', 'analytics-track': 'iphone - index/tab', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100'}]}}, {'kind': 'customsearch#result', 'title': 'iPhone - Wikipedia', 'htmlTitle': '<b>iPhone</b> - Wikipedia', 'link': 'https://en.wikipedia.org/wiki/IPhone', 'displayLink': 'en.wikipedia.org', 'snippet': "iPhone ... For other uses, see iPhone (disambiguation). The iPhone is a line of smartphones developed and marketed by Apple that run iOS, the company's own mobile\xa0...", 'htmlSnippet': '<b>iPhone</b> ... For other uses, see <b>iPhone</b> (disambiguation). The <b>iPhone</b> is a line of smartphones developed and marketed by Apple that run iOS, the company's own mobile ...', 'formattedUrl': 'https://en.wikipedia.org/wiki/IPhone', 'htmlFormattedUrl': 'https://en.wikipedia.org/wiki/<b>IPhone</b>', 'pagemap': {'metatags': [{'referrer': 'origin', 'og:image': 'https://upload.wikimedia.org/wikipedia/en/e/ee/Front_%26_Back_Face_of_iPhone_16_Pro_Max.png', 'theme-color': '#eaecf0', 'og:image:width': '1200', 'og:type': 'website', 'viewport': 'width=device-width, initial-scale=1.0, user-scalable=yes, minimum-scale=0.25, maximum-scale=5.0', 'og:title': 'iPhone - Wikipedia', 'og:image:height': '1423', 'format-detection': 'telephone=no'}], 'hproduct': [{'fn': 'iPhone', 'url': 'apple.com/iphone'}]}}, {'kind': 'customsearch#result', 'title': 'Apple', 'htmlTitle': 'Apple', 'link': 'https://www.apple.com/', 'displayLink': 'www.apple.com', 'snippet': 'Discover the innovative world of Apple and shop everything iPhone, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment,\xa0...', 'htmlSnippet': 'Discover the innovative world of Apple and shop everything <b>iPhone</b>, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, ...', 'formattedUrl': 'https://www.apple.com/', 'htmlFormattedUrl': 'https://www.apple.com/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRU17BvguBEtrzqLvgQkLdknkk8vZsRlqab1b8SC5XiOzqGTdFtArLLMw&s=0', 'width': '150', 'height': '79'}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'analytics-s-bucket-0': 'applestoreww', 'og:image': 'https://www.apple.com/ac/structured-data/images/open_graph_logo.png?202110180743', 'og:type': 'website', 'og:site_name': 'Apple', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'Apple', 'og:description': 'Discover the innovative world of Apple and shop everything iPhone, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, and expert device support.', 'analytics-s-channel': 'homepage', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'og:locale': 'en_US', 'og:url': 'https://www.apple.com/', 'analytics-track': 'Apple - Index/Tab', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/ac/structured-data/images/open_graph_logo.png?202110180743'}]}}, {'kind': 'customsearch#result', 'title': 'Apple iPhone - Walmart.com', 'htmlTitle': 'Apple <b>iPhone</b> - Walmart.com', 'link': 'https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173', 'displayLink': 'www.walmart.com', 'snippet': 'Shop for Apple iPhone at Walmart and save.', 'htmlSnippet': 'Shop for Apple <b>iPhone</b> at Walmart and save.', 'formattedUrl': 'https://www.walmart.com/browse/cell...iphone/1105910_7551331_112717...', 'htmlFormattedUrl': 'https://www.walmart.com/browse/cell...<b>iphone</b>/1105910_7551331_112717...', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRIpWNIuWqABoeCNoSiltxtZvq3G-HeNHvTzQGF05buRP2PsFt9_9FJ_hU&s', 'width': '180', 'height': '180'}], 'metatags': [{'og:image': 'https://i5.walmartimages.com/seo/Total-by-Verizon-Apple-iPhone-12-64GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon_66b2853b-6cb5-4f20-b73a-b60b39b6de44.6b3bf83a920058a47342318925f1dc2b.jpeg?odnHeight=180&odnWidth=180&odnBg=FFFFFF', 'next-head-count': '27', 'fb:app_id': '105223049547814', 'og:type': 'Website', 'og:site_name': 'Walmart.com', 'viewport': 'width=device-width, initial-scale=1.0, minimum-scale=1, interactive-widget=resizes-content', 'og:title': 'Apple iPhone - Walmart.com', 'og:url': 'https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173', 'og:description': 'Shop for Apple iPhone at Walmart and save.'}], 'cse_image': [{'src': 'https://i5.walmartimages.com/seo/Total-by-Verizon-Apple-iPhone-12-64GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon_66b2853b-6cb5-4f20-b73a-b60b39b6de44.6b3bf83a920058a47342318925f1dc2b.jpeg?odnHeight=180&odnWidth=180&odnBg=FFFFFF'}], 'hproduct': [{'fn': 'Your Privacy Choices', 'photo': 'https://i5.walmartimages.com/dfwrs/76316474-d730/k2-_3c5ba298-4f19-46be-9fc3-ac49225d19bd.v1.png'}]}}, {'kind': 'customsearch#result', 'title': 'iPhone - Compare Models - Apple', 'htmlTitle': '<b>iPhone</b> - Compare Models - Apple', 'link': 'https://www.apple.com/iphone/compare/', 'displayLink': 'www.apple.com', 'snippet': 'Compare features and technical specifications for iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, iPhone 16 Plus, iPhone SE, and many more.', 'htmlSnippet': 'Compare features and technical specifications for <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, <b>iPhone</b> 16 Plus, <b>iPhone</b> SE, and many more.', 'formattedUrl': 'https://www.apple.com/iphone/compare/', 'htmlFormattedUrl': 'https://www.apple.com/<b>iphone</b>/compare/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTzLb5dy3cvHFpnrcOVU29mrKsUkOUb4QmCFXw_T_mwVA29b8M2nHk0tUQ&s', 'width': '310', 'height': '163'}], 'BreadcrumbList': [{}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'og:image': 'https://www.apple.com/v/iphone/compare/ah/images/meta/compare__b5xf30djrsia_og.png?202412111328', 'analytics-s-bucket-0': 'applestoreww', 'og:type': 'website', 'og:site_name': 'Apple', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'iPhone - Compare Models', 'og:description': 'Compare features and technical specifications for iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, iPhone 16 Plus, iPhone SE, and many more.', 'analytics-s-channel': 'iphone.tab+other', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac-custom-local-desc-start': 'Compare features and technical specifications for the', 'og:locale': 'en_US', 'ac:tradein-alias': 'tradein-iphone12=slug:model_iphone_12', 'position': '1', 'og:url': 'https://www.apple.com/iphone/compare/', 'ac-custom-local-desc-end': 'and many more.', 'analytics-track': 'iphone - compare', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/v/iphone/compare/ah/images/meta/compare__b5xf30djrsia_og.png?202412111328'}]}}, {'kind': 'customsearch#result', 'title': 'Update your iPhone or iPad - Apple Support', 'htmlTitle': 'Update your <b>iPhone</b> or iPad - Apple Support', 'link': 'https://support.apple.com/en-us/118575', 'displayLink': 'support.apple.com', 'snippet': 'Sep 19, 2024 ... Update your iPhone or iPad wirelessly · Back up your device using iCloud or your computer. · Plug your device into power and connect to the\xa0...', 'htmlSnippet': 'Sep 19, 2024 <b>...</b> Update your <b>iPhone</b> or iPad wirelessly · Back up your device using iCloud or your computer. · Plug your device into power and connect to the ...', 'formattedUrl': 'https://support.apple.com/en-us/118575', 'htmlFormattedUrl': 'https://support.apple.com/en-us/118575', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSafPk1caLY6LV4JEVDvRjg3kmVQamcLheqsPNRq1T3XBDfAjjYR78xl3E&s', 'width': '310', 'height': '163'}], 'Organization': [{'name': 'Apple', 'telephone': '1-800-692-7753'}], 'BreadcrumbList': [{}], 'metatags': [{'og:image': 'https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg', 'og:image:width': '630', 'twitter:card': 'summary_large_image', 'globalnav-search-field[locale]': 'en_US', 'og:site_name': 'Apple Support', 'globalnav-store-key': 'S2A49YFKJF2JAT22K', 'globalnav-search-field[src]': 'globalnav_support', 'og:description': 'Learn how to update your iPhone or iPad to the latest version of iOS or iPadOS.', 'twitter:image': 'https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg', 'globalnav-search-field[placeholder]': 'Search Support', 'twitter:site': '@AppleSupport', 'globalnav-search-field[type]': 'organic', 'globalnav-search-field[page]': 'search', 'og:type': 'article', 'twitter:title': 'Update your iPhone or iPad', 'og:title': 'Update your iPhone or iPad - Apple Support', 'og:image:height': '1200', 'globalnav-search-field[name]': 'q', 'telephone': '1-800-692-7753', 'globalnav-search-field[action]': 'https://support.apple.com/kb/index', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'twitter:description': 'Learn how to update your iPhone or iPad to the latest version of iOS or iPadOS.', 'og:locale': 'en_US', 'name': 'Apple', 'position': '1', 'og:url': 'https://support.apple.com/en-us/118575'}], 'cse_image': [{'src': 'https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg'}]}}, {'kind': 'customsearch#result', 'title': 'Telegram Messenger on the App Store', 'htmlTitle': 'Telegram Messenger on the App Store', 'link': 'https://apps.apple.com/us/app/telegram-messenger/id686449807', 'displayLink': 'apps.apple.com', 'snippet': 'iPhone: Requires iOS 12.0 or later. iPad: Requires iPadOS 12.0 or later. iPod touch: Requires iOS 12.0 or later. Apple Vision: Requires visionOS 1.0 or later\xa0...', 'htmlSnippet': '<b>iPhone</b>: Requires iOS 12.0 or later. iPad: Requires iPadOS 12.0 or later. iPod touch: Requires iOS 12.0 or later. Apple Vision: Requires visionOS 1.0 or later ...', 'formattedUrl': 'https://apps.apple.com/us/app/telegram-messenger/id686449807', 'htmlFormattedUrl': 'https://apps.apple.com/us/app/telegram-messenger/id686449807', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTi86mx0K6hvU0r_kJDP7X1_tuMFhG6srkwXefJd519GTppZyyYuWQrKI4&s', 'width': '310', 'height': '163'}], 'metatags': [{'og:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png', 'og:image:width': '1200', 'twitter:card': 'summary_large_image', 'og:site_name': 'App Store', 'applicable-device': 'pc,mobile', 'og:image:type': 'image/png', 'og:description': 'Pure instant messaging — simple, fast, secure, and synced across all your devices. One of the top 5 most downloaded apps in the world with over 950 million active users.\n\nFAST: Telegram is the fastest messaging app on the market, connecting people via a unique, distributed network of data centers ar…', 'og:image:secure_url': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png', 'twitter:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x600wa.png', 'web-experience-app/config/environment': '%7B%22appVersion%22%3A1%2C%22modulePrefix%22%3A%22web-experience-app%22%2C%22environment%22%3A%22production%22%2C%22rootURL%22%3A%22%2F%22%2C%22locationType%22%3A%22history-hash-router-scroll%22%2C%22historySupportMiddleware%22%3Atrue%2C%22EmberENV%22%3A%7B%22FEATURES%22%3A%7B%7D%2C%22EXTEND_PROTOTYPES%22%3A%7B%22Date%22%3Afalse%7D%2C%22_APPLICATION_TEMPLATE_WRAPPER%22%3Afalse%2C%22_DEFAULT_ASYNC_OBSERVERS%22%3Atrue%2C%22_JQUERY_INTEGRATION%22%3Afalse%2C%22_TEMPLATE_ONLY_GLIMMER_COMPONENTS%22%3Atrue%7D%2C%22APP%22%3A%7B%22PROGRESS_BAR_DELAY%22%3A3000%2C%22CLOCK_INTERVAL%22%3A1000%2C%22LOADING_SPINNER_SPY%22%3Atrue%2C%22BREAKPOINTS%22%3A%7B%22large%22%3A%7B%22min%22%3A1069%2C%22content%22%3A980%7D%2C%22medium%22%3A%7B%22min%22%3A735%2C%22max%22%3A1068%2C%22content%22%3A692%7D%2C%22small%22%3A%7B%22min%22%3A320%2C%22max%22%3A734%2C%22content%22%3A280%7D%7D%2C%22buildVariant%22%3A%22apps%22%2C%22name%22%3A%22web-experience-app%22%2C%22version%22%3A%222450.1.0%2B6799f125%22%7D%2C%22MEDIA_API%22%3A%7B%22token%22%3', 'twitter:image:alt': 'Telegram Messenger on the App\xa0Store', 'twitter:site': '@AppStore', 'og:image:alt': 'Telegram Messenger on the App\xa0Store', 'og:type': 'website', 'twitter:title': 'Telegram Messenger', 'og:title': 'Telegram Messenger', 'og:image:height': '630', 'version': '2450.1.0', 'globalnav-search-suggestions-enabled': 'false', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac-gn-search-suggestions-enabled': 'false', 'twitter:description': 'Pure instant messaging — simple, fast, secure, and synced across all your devices. One of the top 5 most downloaded apps in the world with over 950 million active users.\n\nFAST: Telegram is the fastest messaging app on the market, connecting people via a unique, distributed network of data centers ar…', 'og:locale': 'en_US', 'apple:content_id': '686449807', 'og:url': 'https://apps.apple.com/us/app/telegram-messenger/id686449807'}], 'cse_image': [{'src': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png'}]}}, {'kind': 'customsearch#result', 'title': 'r/iPhone', 'htmlTitle': 'r/<b>iPhone</b>', 'link': 'https://www.reddit.com/r/iphone/', 'displayLink': 'www.reddit.com', 'snippet': "r/iphone: Reddit's little corner for iPhone lovers (and some people who just mildly enjoy it…)", 'htmlSnippet': 'r/<b>iphone</b>: Reddit's little corner for <b>iPhone</b> lovers (and some people who just mildly enjoy it…)', 'formattedUrl': 'https://www.reddit.com/r/iphone/', 'htmlFormattedUrl': 'https://www.reddit.com/r/<b>iphone</b>/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSIdavNM8sk5fHPLXO2OdOBUBDBfHfnuGH6tXC7xcmo_xj1hy-QzHPqdBkx&s', 'width': '227', 'height': '222'}], 'metatags': [{'og:image': 'https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa', 'theme-color': '#000000', 'og:image:width': '256', 'og:type': 'website', 'twitter:card': 'summary', 'twitter:title': 'r/iphone', 'og:site_name': 'Reddit', 'og:title': 'r/iphone', 'og:image:height': '256', 'bingbot': 'noarchive', 'msapplication-navbutton-color': '#000000', 'og:description': 'Reddit’s little corner for iPhone lovers (and some people who just mildly enjoy it…)', 'twitter:image': 'https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa', 'apple-mobile-web-app-status-bar-style': 'black', 'twitter:site': '@reddit', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'apple-mobile-web-app-capable': 'yes', 'og:ttl': '600', 'og:url': 'https://www.reddit.com/r/iphone/'}], 'cse_image': [{'src': 'https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa'}]}}, {'kind': 'customsearch#result', 'title': 'iPhone Repair and Service - Apple Support', 'htmlTitle': '<b>iPhone</b> Repair and Service - Apple Support', 'link': 'https://support.apple.com/iphone/repair', 'displayLink': 'support.apple.com', 'snippet': 'Use our “Get an Estimate” tool to review potential costs if you get service directly from Apple. If you go to another service provider, they can set their own\xa0...', 'htmlSnippet': 'Use our “Get an Estimate” tool to review potential costs if you get service directly from Apple. If you go to another service provider, they can set their own ...', 'formattedUrl': 'https://support.apple.com/iphone/repair', 'htmlFormattedUrl': 'https://support.apple.com/<b>iphone</b>/repair', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTdEyIxqj7ExppNkJKjZtJuqaGqgVBPzm3zYd6LUNtH7u-uJZtEqxfk3Mar&s', 'width': '263', 'height': '192'}], 'Organization': [{'name': 'Apple', 'telephone': '1-800-692-7753'}], 'BreadcrumbList': [{}], 'metatags': [{'og:type': 'article', 'globalnav-search-field[locale]': 'en_US', 'og:site_name': 'Apple Support', 'globalnav-store-key': 'S2A49YFKJF2JAT22K', 'og:title': 'iPhone Repair and Service - Apple Support', 'globalnav-search-field[src]': 'globalnav_support', 'globalnav-search-field[name]': 'q', 'telephone': '1-800-692-7753', 'og:description': 'Need to repair your iPhone? See your service options, their costs by coverage type, and how long they take.', 'globalnav-search-field[placeholder]': 'Search Support', 'globalnav-search-field[action]': 'https://support.apple.com/kb/index', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'og:locale': 'en_US', 'globalnav-search-field[type]': 'organic', 'name': 'Apple', 'globalnav-search-field[page]': 'search', 'position': '1', 'og:url': 'https://support.apple.com/iphone/repair'}], 'cse_image': [{'src': 'https://cdsassets.apple.com/live/7WUAS350/sfaq/SFAQ-header-iphone_2x-gray.png'}]}}, {'kind': 'customsearch#result', 'title': 'WhatsApp Messenger on the App Store', 'htmlTitle': 'WhatsApp Messenger on the App Store', 'link': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997', 'displayLink': 'apps.apple.com', 'snippet': 'Dec 16, 2024 ... This app is available only on the App Store for iPhone, iPad, and Mac. WhatsApp Messenger 12+. Simple. Reliable. Private. WhatsApp Inc. #2 in\xa0...', 'htmlSnippet': 'Dec 16, 2024 <b>...</b> This app is available only on the App Store for <b>iPhone</b>, iPad, and Mac. WhatsApp Messenger 12+. Simple. Reliable. Private. WhatsApp Inc. #2 in ...', 'formattedUrl': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997', 'htmlFormattedUrl': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSgvcpvLlQU9FI1s23H-2B5gelrpNToJqc1vHO_tdCb7vfiagpC5c5E3BM&s', 'width': '310', 'height': '163'}], 'metatags': [{'og:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png', 'og:image:width': '1200', 'twitter:card': 'summary_large_image', 'og:site_name': 'App Store', 'applicable-device': 'pc,mobile', 'og:image:type': 'image/png', 'og:description': 'With WhatsApp for Mac, you can conveniently sync all your chats to your computer. Message privately, make calls and share files with your friends, family and colleagues.', 'og:image:secure_url': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png', 'twitter:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x600wa.png', 'web-experience-app/config/environment': '%7B%22appVersion%22%3A1%2C%22modulePrefix%22%3A%22web-experience-app%22%2C%22environment%22%3A%22production%22%2C%22rootURL%22%3A%22%2F%22%2C%22locationType%22%3A%22history-hash-router-scroll%22%2C%22historySupportMiddleware%22%3Atrue%2C%22EmberENV%22%3A%7B%22FEATURES%22%3A%7B%7D%2C%22EXTEND_PROTOTYPES%22%3A%7B%22Date%22%3Afalse%7D%2C%22_APPLICATION_TEMPLATE_WRAPPER%22%3Afalse%2C%22_DEFAULT_ASYNC_OBSERVERS%22%3Atrue%2C%22_JQUERY_INTEGRATION%22%3Afalse%2C%22_TEMPLATE_ONLY_GLIMMER_COMPONENTS%22%3Atrue%7D%2C%22APP%22%3A%7B%22PROGRESS_BAR_DELAY%22%3A3000%2C%22CLOCK_INTERVAL%22%3A1000%2C%22LOADING_SPINNER_SPY%22%3Atrue%2C%22BREAKPOINTS%22%3A%7B%22large%22%3A%7B%22min%22%3A1069%2C%22content%22%3A980%7D%2C%22medium%22%3A%7B%22min%22%3A735%2C%22max%22%3A1068%2C%22content%22%3A692%7D%2C%22small%22%3A%7B%22min%22%3A320%2C%22max%22%3A734%2C%22content%22%3A280%7D%7D%2C%22buildVariant%22%3A%22apps%22%2C%22name%22%3A%22web-experience-app%22%2C%22version%22%3A%222450.1.0%2B6799f125%22%7D%2C%22MEDIA_API%22%3A%7B%22token%22%3', 'twitter:image:alt': 'WhatsApp Messenger on the App\xa0Store', 'twitter:site': '@AppStore', 'og:image:alt': 'WhatsApp Messenger on the App\xa0Store', 'og:type': 'website', 'twitter:title': 'WhatsApp Messenger', 'og:title': 'WhatsApp Messenger', 'og:image:height': '630', 'version': '2450.1.0', 'globalnav-search-suggestions-enabled': 'false', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac-gn-search-suggestions-enabled': 'false', 'twitter:description': 'With WhatsApp for Mac, you can conveniently sync all your chats to your computer. Message privately, make calls and share files with your friends, family and colleagues.', 'og:locale': 'en_US', 'apple:content_id': '310633997', 'og:url': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997'}], 'cse_image': [{'src': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png'}]}}]} LastBuildDate: None Items: [{'kind': 'customsearch#result', 'title': 'iPhone - Apple', 'htmlTitle': '<b>iPhone</b> - Apple', 'link': 'https://www.apple.com/iphone/', 'displayLink': 'www.apple.com', 'snippet': 'Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.', 'htmlSnippet': 'Designed for Apple Intelligence. Discover the new <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, and <b>iPhone</b> 16 Plus.', 'formattedUrl': 'https://www.apple.com/iphone/', 'htmlFormattedUrl': 'https://www.apple.com/<b>iphone</b>/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSTP05LrdG82MHIYno9vsxK6v__4F7GDtXjDnOCzLzKXdMSrHVkT5J4BNA&s', 'width': '310', 'height': '163'}], 'BreadcrumbList': [{}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'og:image': 'https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100', 'analytics-s-bucket-0': 'applestoreww', 'og:type': 'website', 'twitter:card': 'summary_large_image', 'og:site_name': 'Apple', 'al:ios:app_name': 'Apple Store', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'iPhone', 'al:ios:url': 'https://www.apple.com/us/xc/iphone?cid=AOS_ASA', 'og:description': 'Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.', 'al:ios:app_store_id': '375380948', 'analytics-s-channel': 'iphone', 'twitter:site': '@Apple', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac:pricing-alias': 'iphone-16-pro=IPHONE16PRO', 'og:locale': 'en_US', 'ac:tradein-alias': 'tradein-iphone12=slug:model_iphone_12', 'position': '1', 'og:url': 'https://www.apple.com/iphone/', 'analytics-track': 'iphone - index/tab', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100'}]}}, {'kind': 'customsearch#result', 'title': 'iPhone - Wikipedia', 'htmlTitle': '<b>iPhone</b> - Wikipedia', 'link': 'https://en.wikipedia.org/wiki/IPhone', 'displayLink': 'en.wikipedia.org', 'snippet': "iPhone ... For other uses, see iPhone (disambiguation). The iPhone is a line of smartphones developed and marketed by Apple that run iOS, the company's own mobile\xa0...", 'htmlSnippet': '<b>iPhone</b> ... For other uses, see <b>iPhone</b> (disambiguation). The <b>iPhone</b> is a line of smartphones developed and marketed by Apple that run iOS, the company's own mobile ...', 'formattedUrl': 'https://en.wikipedia.org/wiki/IPhone', 'htmlFormattedUrl': 'https://en.wikipedia.org/wiki/<b>IPhone</b>', 'pagemap': {'metatags': [{'referrer': 'origin', 'og:image': 'https://upload.wikimedia.org/wikipedia/en/e/ee/Front_%26_Back_Face_of_iPhone_16_Pro_Max.png', 'theme-color': '#eaecf0', 'og:image:width': '1200', 'og:type': 'website', 'viewport': 'width=device-width, initial-scale=1.0, user-scalable=yes, minimum-scale=0.25, maximum-scale=5.0', 'og:title': 'iPhone - Wikipedia', 'og:image:height': '1423', 'format-detection': 'telephone=no'}], 'hproduct': [{'fn': 'iPhone', 'url': 'apple.com/iphone'}]}}, {'kind': 'customsearch#result', 'title': 'Apple', 'htmlTitle': 'Apple', 'link': 'https://www.apple.com/', 'displayLink': 'www.apple.com', 'snippet': 'Discover the innovative world of Apple and shop everything iPhone, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment,\xa0...', 'htmlSnippet': 'Discover the innovative world of Apple and shop everything <b>iPhone</b>, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, ...', 'formattedUrl': 'https://www.apple.com/', 'htmlFormattedUrl': 'https://www.apple.com/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRU17BvguBEtrzqLvgQkLdknkk8vZsRlqab1b8SC5XiOzqGTdFtArLLMw&s=0', 'width': '150', 'height': '79'}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'analytics-s-bucket-0': 'applestoreww', 'og:image': 'https://www.apple.com/ac/structured-data/images/open_graph_logo.png?202110180743', 'og:type': 'website', 'og:site_name': 'Apple', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'Apple', 'og:description': 'Discover the innovative world of Apple and shop everything iPhone, iPad, Apple Watch, Mac, and Apple TV, plus explore accessories, entertainment, and expert device support.', 'analytics-s-channel': 'homepage', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'og:locale': 'en_US', 'og:url': 'https://www.apple.com/', 'analytics-track': 'Apple - Index/Tab', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/ac/structured-data/images/open_graph_logo.png?202110180743'}]}}, {'kind': 'customsearch#result', 'title': 'Apple iPhone - Walmart.com', 'htmlTitle': 'Apple <b>iPhone</b> - Walmart.com', 'link': 'https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173', 'displayLink': 'www.walmart.com', 'snippet': 'Shop for Apple iPhone at Walmart and save.', 'htmlSnippet': 'Shop for Apple <b>iPhone</b> at Walmart and save.', 'formattedUrl': 'https://www.walmart.com/browse/cell...iphone/1105910_7551331_112717...', 'htmlFormattedUrl': 'https://www.walmart.com/browse/cell...<b>iphone</b>/1105910_7551331_112717...', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRIpWNIuWqABoeCNoSiltxtZvq3G-HeNHvTzQGF05buRP2PsFt9_9FJ_hU&s', 'width': '180', 'height': '180'}], 'metatags': [{'og:image': 'https://i5.walmartimages.com/seo/Total-by-Verizon-Apple-iPhone-12-64GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon_66b2853b-6cb5-4f20-b73a-b60b39b6de44.6b3bf83a920058a47342318925f1dc2b.jpeg?odnHeight=180&odnWidth=180&odnBg=FFFFFF', 'next-head-count': '27', 'fb:app_id': '105223049547814', 'og:type': 'Website', 'og:site_name': 'Walmart.com', 'viewport': 'width=device-width, initial-scale=1.0, minimum-scale=1, interactive-widget=resizes-content', 'og:title': 'Apple iPhone - Walmart.com', 'og:url': 'https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173', 'og:description': 'Shop for Apple iPhone at Walmart and save.'}], 'cse_image': [{'src': 'https://i5.walmartimages.com/seo/Total-by-Verizon-Apple-iPhone-12-64GB-Black-Prepaid-Smartphone-Locked-to-Total-by-Verizon_66b2853b-6cb5-4f20-b73a-b60b39b6de44.6b3bf83a920058a47342318925f1dc2b.jpeg?odnHeight=180&odnWidth=180&odnBg=FFFFFF'}], 'hproduct': [{'fn': 'Your Privacy Choices', 'photo': 'https://i5.walmartimages.com/dfwrs/76316474-d730/k2-_3c5ba298-4f19-46be-9fc3-ac49225d19bd.v1.png'}]}}, {'kind': 'customsearch#result', 'title': 'iPhone - Compare Models - Apple', 'htmlTitle': '<b>iPhone</b> - Compare Models - Apple', 'link': 'https://www.apple.com/iphone/compare/', 'displayLink': 'www.apple.com', 'snippet': 'Compare features and technical specifications for iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, iPhone 16 Plus, iPhone SE, and many more.', 'htmlSnippet': 'Compare features and technical specifications for <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, <b>iPhone</b> 16 Plus, <b>iPhone</b> SE, and many more.', 'formattedUrl': 'https://www.apple.com/iphone/compare/', 'htmlFormattedUrl': 'https://www.apple.com/<b>iphone</b>/compare/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTzLb5dy3cvHFpnrcOVU29mrKsUkOUb4QmCFXw_T_mwVA29b8M2nHk0tUQ&s', 'width': '310', 'height': '163'}], 'BreadcrumbList': [{}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'og:image': 'https://www.apple.com/v/iphone/compare/ah/images/meta/compare__b5xf30djrsia_og.png?202412111328', 'analytics-s-bucket-0': 'applestoreww', 'og:type': 'website', 'og:site_name': 'Apple', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'iPhone - Compare Models', 'og:description': 'Compare features and technical specifications for iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, iPhone 16 Plus, iPhone SE, and many more.', 'analytics-s-channel': 'iphone.tab+other', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac-custom-local-desc-start': 'Compare features and technical specifications for the', 'og:locale': 'en_US', 'ac:tradein-alias': 'tradein-iphone12=slug:model_iphone_12', 'position': '1', 'og:url': 'https://www.apple.com/iphone/compare/', 'ac-custom-local-desc-end': 'and many more.', 'analytics-track': 'iphone - compare', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/v/iphone/compare/ah/images/meta/compare__b5xf30djrsia_og.png?202412111328'}]}}, {'kind': 'customsearch#result', 'title': 'Update your iPhone or iPad - Apple Support', 'htmlTitle': 'Update your <b>iPhone</b> or iPad - Apple Support', 'link': 'https://support.apple.com/en-us/118575', 'displayLink': 'support.apple.com', 'snippet': 'Sep 19, 2024 ... Update your iPhone or iPad wirelessly · Back up your device using iCloud or your computer. · Plug your device into power and connect to the\xa0...', 'htmlSnippet': 'Sep 19, 2024 <b>...</b> Update your <b>iPhone</b> or iPad wirelessly · Back up your device using iCloud or your computer. · Plug your device into power and connect to the ...', 'formattedUrl': 'https://support.apple.com/en-us/118575', 'htmlFormattedUrl': 'https://support.apple.com/en-us/118575', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSafPk1caLY6LV4JEVDvRjg3kmVQamcLheqsPNRq1T3XBDfAjjYR78xl3E&s', 'width': '310', 'height': '163'}], 'Organization': [{'name': 'Apple', 'telephone': '1-800-692-7753'}], 'BreadcrumbList': [{}], 'metatags': [{'og:image': 'https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg', 'og:image:width': '630', 'twitter:card': 'summary_large_image', 'globalnav-search-field[locale]': 'en_US', 'og:site_name': 'Apple Support', 'globalnav-store-key': 'S2A49YFKJF2JAT22K', 'globalnav-search-field[src]': 'globalnav_support', 'og:description': 'Learn how to update your iPhone or iPad to the latest version of iOS or iPadOS.', 'twitter:image': 'https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg', 'globalnav-search-field[placeholder]': 'Search Support', 'twitter:site': '@AppleSupport', 'globalnav-search-field[type]': 'organic', 'globalnav-search-field[page]': 'search', 'og:type': 'article', 'twitter:title': 'Update your iPhone or iPad', 'og:title': 'Update your iPhone or iPad - Apple Support', 'og:image:height': '1200', 'globalnav-search-field[name]': 'q', 'telephone': '1-800-692-7753', 'globalnav-search-field[action]': 'https://support.apple.com/kb/index', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'twitter:description': 'Learn how to update your iPhone or iPad to the latest version of iOS or iPadOS.', 'og:locale': 'en_US', 'name': 'Apple', 'position': '1', 'og:url': 'https://support.apple.com/en-us/118575'}], 'cse_image': [{'src': 'https://cdsassets.apple.com/live/7WUAS350/images/social/support-app-hero/update-software-social-card.jpg'}]}}, {'kind': 'customsearch#result', 'title': 'Telegram Messenger on the App Store', 'htmlTitle': 'Telegram Messenger on the App Store', 'link': 'https://apps.apple.com/us/app/telegram-messenger/id686449807', 'displayLink': 'apps.apple.com', 'snippet': 'iPhone: Requires iOS 12.0 or later. iPad: Requires iPadOS 12.0 or later. iPod touch: Requires iOS 12.0 or later. Apple Vision: Requires visionOS 1.0 or later\xa0...', 'htmlSnippet': '<b>iPhone</b>: Requires iOS 12.0 or later. iPad: Requires iPadOS 12.0 or later. iPod touch: Requires iOS 12.0 or later. Apple Vision: Requires visionOS 1.0 or later ...', 'formattedUrl': 'https://apps.apple.com/us/app/telegram-messenger/id686449807', 'htmlFormattedUrl': 'https://apps.apple.com/us/app/telegram-messenger/id686449807', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTi86mx0K6hvU0r_kJDP7X1_tuMFhG6srkwXefJd519GTppZyyYuWQrKI4&s', 'width': '310', 'height': '163'}], 'metatags': [{'og:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png', 'og:image:width': '1200', 'twitter:card': 'summary_large_image', 'og:site_name': 'App Store', 'applicable-device': 'pc,mobile', 'og:image:type': 'image/png', 'og:description': 'Pure instant messaging — simple, fast, secure, and synced across all your devices. One of the top 5 most downloaded apps in the world with over 950 million active users.\n\nFAST: Telegram is the fastest messaging app on the market, connecting people via a unique, distributed network of data centers ar…', 'og:image:secure_url': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png', 'twitter:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x600wa.png', 'web-experience-app/config/environment': '%7B%22appVersion%22%3A1%2C%22modulePrefix%22%3A%22web-experience-app%22%2C%22environment%22%3A%22production%22%2C%22rootURL%22%3A%22%2F%22%2C%22locationType%22%3A%22history-hash-router-scroll%22%2C%22historySupportMiddleware%22%3Atrue%2C%22EmberENV%22%3A%7B%22FEATURES%22%3A%7B%7D%2C%22EXTEND_PROTOTYPES%22%3A%7B%22Date%22%3Afalse%7D%2C%22_APPLICATION_TEMPLATE_WRAPPER%22%3Afalse%2C%22_DEFAULT_ASYNC_OBSERVERS%22%3Atrue%2C%22_JQUERY_INTEGRATION%22%3Afalse%2C%22_TEMPLATE_ONLY_GLIMMER_COMPONENTS%22%3Atrue%7D%2C%22APP%22%3A%7B%22PROGRESS_BAR_DELAY%22%3A3000%2C%22CLOCK_INTERVAL%22%3A1000%2C%22LOADING_SPINNER_SPY%22%3Atrue%2C%22BREAKPOINTS%22%3A%7B%22large%22%3A%7B%22min%22%3A1069%2C%22content%22%3A980%7D%2C%22medium%22%3A%7B%22min%22%3A735%2C%22max%22%3A1068%2C%22content%22%3A692%7D%2C%22small%22%3A%7B%22min%22%3A320%2C%22max%22%3A734%2C%22content%22%3A280%7D%7D%2C%22buildVariant%22%3A%22apps%22%2C%22name%22%3A%22web-experience-app%22%2C%22version%22%3A%222450.1.0%2B6799f125%22%7D%2C%22MEDIA_API%22%3A%7B%22token%22%3', 'twitter:image:alt': 'Telegram Messenger on the App\xa0Store', 'twitter:site': '@AppStore', 'og:image:alt': 'Telegram Messenger on the App\xa0Store', 'og:type': 'website', 'twitter:title': 'Telegram Messenger', 'og:title': 'Telegram Messenger', 'og:image:height': '630', 'version': '2450.1.0', 'globalnav-search-suggestions-enabled': 'false', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac-gn-search-suggestions-enabled': 'false', 'twitter:description': 'Pure instant messaging — simple, fast, secure, and synced across all your devices. One of the top 5 most downloaded apps in the world with over 950 million active users.\n\nFAST: Telegram is the fastest messaging app on the market, connecting people via a unique, distributed network of data centers ar…', 'og:locale': 'en_US', 'apple:content_id': '686449807', 'og:url': 'https://apps.apple.com/us/app/telegram-messenger/id686449807'}], 'cse_image': [{'src': 'https://is1-ssl.mzstatic.com/image/thumb/Purple211/v4/26/eb/74/26eb74f2-a335-ba89-d02f-5566d1af3433/AppIconLLC-0-0-1x_U007emarketing-0-8-0-0-85-220.png/1200x630wa.png'}]}}, {'kind': 'customsearch#result', 'title': 'r/iPhone', 'htmlTitle': 'r/<b>iPhone</b>', 'link': 'https://www.reddit.com/r/iphone/', 'displayLink': 'www.reddit.com', 'snippet': "r/iphone: Reddit's little corner for iPhone lovers (and some people who just mildly enjoy it…)", 'htmlSnippet': 'r/<b>iphone</b>: Reddit's little corner for <b>iPhone</b> lovers (and some people who just mildly enjoy it…)', 'formattedUrl': 'https://www.reddit.com/r/iphone/', 'htmlFormattedUrl': 'https://www.reddit.com/r/<b>iphone</b>/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSIdavNM8sk5fHPLXO2OdOBUBDBfHfnuGH6tXC7xcmo_xj1hy-QzHPqdBkx&s', 'width': '227', 'height': '222'}], 'metatags': [{'og:image': 'https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa', 'theme-color': '#000000', 'og:image:width': '256', 'og:type': 'website', 'twitter:card': 'summary', 'twitter:title': 'r/iphone', 'og:site_name': 'Reddit', 'og:title': 'r/iphone', 'og:image:height': '256', 'bingbot': 'noarchive', 'msapplication-navbutton-color': '#000000', 'og:description': 'Reddit’s little corner for iPhone lovers (and some people who just mildly enjoy it…)', 'twitter:image': 'https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa', 'apple-mobile-web-app-status-bar-style': 'black', 'twitter:site': '@reddit', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'apple-mobile-web-app-capable': 'yes', 'og:ttl': '600', 'og:url': 'https://www.reddit.com/r/iphone/'}], 'cse_image': [{'src': 'https://styles.redditmedia.com/t5_2qh2b/styles/communityIcon_ig9nr9020vnb1.jpg?format=pjpg&s=d099cbfa5c87f941a1e65a58a52a18047f151eaa'}]}}, {'kind': 'customsearch#result', 'title': 'iPhone Repair and Service - Apple Support', 'htmlTitle': '<b>iPhone</b> Repair and Service - Apple Support', 'link': 'https://support.apple.com/iphone/repair', 'displayLink': 'support.apple.com', 'snippet': 'Use our “Get an Estimate” tool to review potential costs if you get service directly from Apple. If you go to another service provider, they can set their own\xa0...', 'htmlSnippet': 'Use our “Get an Estimate” tool to review potential costs if you get service directly from Apple. If you go to another service provider, they can set their own ...', 'formattedUrl': 'https://support.apple.com/iphone/repair', 'htmlFormattedUrl': 'https://support.apple.com/<b>iphone</b>/repair', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTdEyIxqj7ExppNkJKjZtJuqaGqgVBPzm3zYd6LUNtH7u-uJZtEqxfk3Mar&s', 'width': '263', 'height': '192'}], 'Organization': [{'name': 'Apple', 'telephone': '1-800-692-7753'}], 'BreadcrumbList': [{}], 'metatags': [{'og:type': 'article', 'globalnav-search-field[locale]': 'en_US', 'og:site_name': 'Apple Support', 'globalnav-store-key': 'S2A49YFKJF2JAT22K', 'og:title': 'iPhone Repair and Service - Apple Support', 'globalnav-search-field[src]': 'globalnav_support', 'globalnav-search-field[name]': 'q', 'telephone': '1-800-692-7753', 'og:description': 'Need to repair your iPhone? See your service options, their costs by coverage type, and how long they take.', 'globalnav-search-field[placeholder]': 'Search Support', 'globalnav-search-field[action]': 'https://support.apple.com/kb/index', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'og:locale': 'en_US', 'globalnav-search-field[type]': 'organic', 'name': 'Apple', 'globalnav-search-field[page]': 'search', 'position': '1', 'og:url': 'https://support.apple.com/iphone/repair'}], 'cse_image': [{'src': 'https://cdsassets.apple.com/live/7WUAS350/sfaq/SFAQ-header-iphone_2x-gray.png'}]}}, {'kind': 'customsearch#result', 'title': 'WhatsApp Messenger on the App Store', 'htmlTitle': 'WhatsApp Messenger on the App Store', 'link': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997', 'displayLink': 'apps.apple.com', 'snippet': 'Dec 16, 2024 ... This app is available only on the App Store for iPhone, iPad, and Mac. WhatsApp Messenger 12+. Simple. Reliable. Private. WhatsApp Inc. #2 in\xa0...', 'htmlSnippet': 'Dec 16, 2024 <b>...</b> This app is available only on the App Store for <b>iPhone</b>, iPad, and Mac. WhatsApp Messenger 12+. Simple. Reliable. Private. WhatsApp Inc. #2 in ...', 'formattedUrl': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997', 'htmlFormattedUrl': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSgvcpvLlQU9FI1s23H-2B5gelrpNToJqc1vHO_tdCb7vfiagpC5c5E3BM&s', 'width': '310', 'height': '163'}], 'metatags': [{'og:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png', 'og:image:width': '1200', 'twitter:card': 'summary_large_image', 'og:site_name': 'App Store', 'applicable-device': 'pc,mobile', 'og:image:type': 'image/png', 'og:description': 'With WhatsApp for Mac, you can conveniently sync all your chats to your computer. Message privately, make calls and share files with your friends, family and colleagues.', 'og:image:secure_url': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png', 'twitter:image': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x600wa.png', 'web-experience-app/config/environment': '%7B%22appVersion%22%3A1%2C%22modulePrefix%22%3A%22web-experience-app%22%2C%22environment%22%3A%22production%22%2C%22rootURL%22%3A%22%2F%22%2C%22locationType%22%3A%22history-hash-router-scroll%22%2C%22historySupportMiddleware%22%3Atrue%2C%22EmberENV%22%3A%7B%22FEATURES%22%3A%7B%7D%2C%22EXTEND_PROTOTYPES%22%3A%7B%22Date%22%3Afalse%7D%2C%22_APPLICATION_TEMPLATE_WRAPPER%22%3Afalse%2C%22_DEFAULT_ASYNC_OBSERVERS%22%3Atrue%2C%22_JQUERY_INTEGRATION%22%3Afalse%2C%22_TEMPLATE_ONLY_GLIMMER_COMPONENTS%22%3Atrue%7D%2C%22APP%22%3A%7B%22PROGRESS_BAR_DELAY%22%3A3000%2C%22CLOCK_INTERVAL%22%3A1000%2C%22LOADING_SPINNER_SPY%22%3Atrue%2C%22BREAKPOINTS%22%3A%7B%22large%22%3A%7B%22min%22%3A1069%2C%22content%22%3A980%7D%2C%22medium%22%3A%7B%22min%22%3A735%2C%22max%22%3A1068%2C%22content%22%3A692%7D%2C%22small%22%3A%7B%22min%22%3A320%2C%22max%22%3A734%2C%22content%22%3A280%7D%7D%2C%22buildVariant%22%3A%22apps%22%2C%22name%22%3A%22web-experience-app%22%2C%22version%22%3A%222450.1.0%2B6799f125%22%7D%2C%22MEDIA_API%22%3A%7B%22token%22%3', 'twitter:image:alt': 'WhatsApp Messenger on the App\xa0Store', 'twitter:site': '@AppStore', 'og:image:alt': 'WhatsApp Messenger on the App\xa0Store', 'og:type': 'website', 'twitter:title': 'WhatsApp Messenger', 'og:title': 'WhatsApp Messenger', 'og:image:height': '630', 'version': '2450.1.0', 'globalnav-search-suggestions-enabled': 'false', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac-gn-search-suggestions-enabled': 'false', 'twitter:description': 'With WhatsApp for Mac, you can conveniently sync all your chats to your computer. Message privately, make calls and share files with your friends, family and colleagues.', 'og:locale': 'en_US', 'apple:content_id': '310633997', 'og:url': 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997'}], 'cse_image': [{'src': 'https://is1-ssl.mzstatic.com/image/thumb/Purple221/v4/d6/08/d5/d608d5e3-8b65-bb2f-b652-30c044f9efe6/AppIconCatalystRelease-0-0-2x_U007euniversal-0-0-0-4-0-0-0-85-220.png/1200x630wa.png'}]}}] First Item: {'kind': 'customsearch#result', 'title': 'iPhone - Apple', 'htmlTitle': '<b>iPhone</b> - Apple', 'link': 'https://www.apple.com/iphone/', 'displayLink': 'www.apple.com', 'snippet': 'Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.', 'htmlSnippet': 'Designed for Apple Intelligence. Discover the new <b>iPhone</b> 16 Pro, <b>iPhone</b> 16 Pro Max, <b>iPhone</b> 16, and <b>iPhone</b> 16 Plus.', 'formattedUrl': 'https://www.apple.com/iphone/', 'htmlFormattedUrl': 'https://www.apple.com/<b>iphone</b>/', 'pagemap': {'cse_thumbnail': [{'src': 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcSTP05LrdG82MHIYno9vsxK6v__4F7GDtXjDnOCzLzKXdMSrHVkT5J4BNA&s', 'width': '310', 'height': '163'}], 'BreadcrumbList': [{}], 'metatags': [{'analytics-s-bucket-1': 'applestoreww', 'og:image': 'https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100', 'analytics-s-bucket-0': 'applestoreww', 'og:type': 'website', 'twitter:card': 'summary_large_image', 'og:site_name': 'Apple', 'al:ios:app_name': 'Apple Store', 'globalnav-store-key': 'SFX9YPYY9PPXCU9KH', 'og:title': 'iPhone', 'al:ios:url': 'https://www.apple.com/us/xc/iphone?cid=AOS_ASA', 'og:description': 'Designed for Apple Intelligence. Discover the new iPhone 16 Pro, iPhone 16 Pro Max, iPhone 16, and iPhone 16 Plus.', 'al:ios:app_store_id': '375380948', 'analytics-s-channel': 'iphone', 'twitter:site': '@Apple', 'viewport': 'width=device-width, initial-scale=1, viewport-fit=cover', 'ac:pricing-alias': 'iphone-16-pro=IPHONE16PRO', 'og:locale': 'en_US', 'ac:tradein-alias': 'tradein-iphone12=slug:model_iphone_12', 'position': '1', 'og:url': 'https://www.apple.com/iphone/', 'analytics-track': 'iphone - index/tab', 'analytics-s-bucket-2': 'applestoreww'}], 'cse_image': [{'src': 'https://www.apple.com/v/iphone/home/by/images/meta/iphone__kqge21l9n26q_og.png?202412181100'}]}} title of First Item: iPhone - Apple Link of First Item: https://www.apple.com/iphone/
import requests
# Define the search query term
query = 'IPhone'
# Initialize an empty list to store search results
search_result = []
# Loop through 10 pages of search results (Google Custom Search API returns 10 results per page)
for num in range(10):
# Construct the API URL with the required parameters: API key, search engine ID, query, and start index
google_open_api = f'https://www.googleapis.com/customsearch/v1?key={GOOGLE_API_KEY}&cx={GOOGLE_CX}&q={query}&start={num*10+1}'
# Send a GET request to the Google Custom Search API
res = requests.get(google_open_api)
# Check if the response status code indicates success
if res.status_code == 200:
# Parse the JSON response
data = res.json()
# Iterate over the 'items' in the response data to extract search results
for index, item in enumerate(data['items']):
# Append the search result to the list, including rank, title, and link
search_result.append([num*10+index + 1, item['title'], item['link']])
else:
# Print an error message if the API request fails
print("Error Code:", res.status_code)
# Print all collected search results
print(search_result)
[[1, 'iPhone - Apple', 'https://www.apple.com/iphone/'], [2, 'iPhone - Wikipedia', 'https://en.wikipedia.org/wiki/IPhone'], [3, 'Apple', 'https://www.apple.com/'], [4, 'Update your iPhone or iPad - Apple Support', 'https://support.apple.com/en-us/118575'], [5, 'iPhone - Compare Models - Apple', 'https://www.apple.com/iphone/compare/'], [6, 'Apple iPhone - Walmart.com', 'https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173'], [7, 'r/iPhone', 'https://www.reddit.com/r/iphone/'], [8, 'Telegram Messenger on the App Store', 'https://apps.apple.com/us/app/telegram-messenger/id686449807'], [9, "Use parental controls on your child's iPhone or iPad - Apple Support", 'https://support.apple.com/en-us/105121'], [10, 'WhatsApp Messenger on the App Store', 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997'], [11, 'All iPhone - Best Buy', 'https://www.bestbuy.com/site/iphone/all-iphone/pcmcat1683750935583.c?id=pcmcat1683750935583'], [12, 'Zoom Workplace on the App Store', 'https://apps.apple.com/us/app/zoom-workplace/id546505307'], [13, 'IPPAWARDS | iPhone Photography Awards – Celebrating the ...', 'https://www.ippawards.com/'], [14, 'Amazon Alexa on the App Store', 'https://apps.apple.com/us/app/amazon-alexa/id944011620'], [15, 'Iphone - Amazon.com', 'https://www.amazon.com/iphone/s?k=iphone'], [16, 'Flickr on the App Store', 'https://apps.apple.com/us/app/flickr/id328407587'], [17, 'New Apple iPhones for Sale: See Pricing & More or Buy Today | T ...', 'https://www.t-mobile.com/cell-phones/brand/apple'], [18, 'Facebook on the App Store', 'https://apps.apple.com/us/app/facebook/id284882215'], [19, 'Find Devices - Apple iCloud', 'https://www.icloud.com/find/'], [20, 'Instagram on the App Store', 'https://apps.apple.com/us/app/instagram/id389801252'], [21, 'Building a giant working iPhone - YouTube', 'https://www.youtube.com/watch?v=o8jFJAtVyr8'], [22, 'BookBook vol. 2 for iPhone | Leather wallet case with removable shell', 'https://www.twelvesouth.com/products/bookbook-vol-2-for-iphone'], [23, '22 New Things Your iPhone Can Do in iOS 18.2 - MacRumors', 'https://www.macrumors.com/2024/12/23/new-things-your-iphone-can-do-in-ios-18-2/'], [24, 'SAP Concur Mobile App User Resources - iPhone | SAP Concur ...', 'https://www.concurtraining.com/toolkit/en/mobile/iphone/end-user'], [25, 'Duo Mobile on iOS - Guide to Two-Factor Authentication · Duo Security', 'https://guide.duo.com/iphone'], [26, "Check your device's coverage", 'https://checkcoverage.apple.com/'], [27, 'iOS 18 - Apple Developer', 'https://developer.apple.com/ios/'], [28, 'AirPods Hearing Test not showing on iPhone - Apple Community', 'https://discussions.apple.com/thread/255767114'], [29, 'How to: Encrypt Your iPhone | Surveillance Self-Defense', 'https://ssd.eff.org/module/how-encrypt-your-iphone'], [30, 'The iOS VoIP App •• iPhone User Manual | 3CX', 'https://www.3cx.com/user-manual/installation-iphone/'], [31, 'FBI Warns iPhone And Android Users—Stop Sending Texts', 'https://www.forbes.com/sites/zakdoffman/2024/12/06/fbi-warns-iphone-and-android-users-stop-sending-texts/'], [32, 'Apple iPhone Repair Help: Learn How to Fix It Yourself.', 'https://www.ifixit.com/Device/iPhone'], [33, 'iPhone | The Guardian', 'https://www.theguardian.com/technology/iphone'], [34, 'iPhone 16/16 Pro Review: Times Have Changed! - YouTube', 'https://www.youtube.com/watch?v=MRtg6A1f2Ko'], [35, 'MFi Program', 'https://mfi.apple.com/'], [36, 'iPhone Photography School | iPhone Photography Online Courses', 'https://iphonephotographyschool.com/'], [37, 'Apple iPhone | endoflife.date', 'https://endoflife.date/iphone'], [38, 'iPhone Cases – CASETiFY', 'https://www.casetify.com/iphone-cases'], [39, 'How to move chats from an Android device to an iPhone | WhatsApp ...', 'https://faq.whatsapp.com/686469079565350'], [40, 'Apple iPhone: Shop Apple Smartphones | Verizon', 'https://www.verizon.com/smartphones/apple/'], [41, 'Best iPhone in 2024: Which Apple Phone Should You Buy? - CNET', 'https://www.cnet.com/tech/mobile/best-iphone/'], [42, 'Download Epic Games for iOS & iPhone - Epic Games Store', 'https://store.epicgames.com/en-US/mobile/ios'], [43, 'Certified Refurbished iPhone | Back Market', 'https://www.backmarket.com/en-us/l/iphone/e8724fea-197e-4815-85ce-21b8068020cc'], [44, 'Choose an iPhone / IPSW Downloads', 'https://ipsw.me/product/iPhone'], [45, 'iPhone Skins » dbrand', 'https://dbrand.com/shop/devices/iphone-skins'], [46, "MacRumors Buyer's Guide: Know When to Buy iPhone, Mac, iPad", 'https://buyersguide.macrumors.com/'], [47, 'Download the Gemini app for iPhone today', 'https://blog.google/products/gemini/gemini-iphone-app/'], [48, 'Accept payments with Tap to Pay on iPhone | Square Support ...', 'https://squareup.com/help/us/en/article/7786-get-started-with-tap-to-pay-on-iphone'], [49, "What's the Best iPhone to Buy or Avoid Right Now? (2024) | WIRED", 'https://www.wired.com/gallery/iphone-buying-guide/'], [50, 'Best iPhones 2024: Which iPhone model should you buy? | ZDNET', 'https://www.zdnet.com/article/best-iphone/'], [51, 'New Apple iPhones, including iPhone 16: Get Prices & Buy Now ...', 'https://www.att.com/buy/phones/browse/apple/'], [52, 'Clicks Keyboard for iPhone', 'https://www.clicks.tech/'], [53, 'iPhone - 2nd Hand Devices | CompAsia Malaysia', 'https://compasia.my/collections/iphone'], [54, 'The Ultimate Guide To iPhone Resolutions', 'https://www.paintcodeapp.com/news/ultimate-guide-to-iphone-resolutions'], [55, 'Apple iPhone 12 Prepaid | Price, Specs & Deals | Metro by T-Moblie', 'https://www.metrobyt-mobile.com/cell-phone/apple-iphone-12'], [56, 'How to Play Fortnite on iPhone iOS or iPad', 'http://www.fortnite.com/mobile/ios?lang=en-US'], [57, 'How the U.S. Lost Out on iPhone Work', 'https://www.nytimes.com/2012/01/22/business/apple-america-and-a-squeezed-middle-class.html'], [58, 'iPhones for Sale - New & Used iPhones at Great Prices - eBay', 'https://www.ebay.com/b/Apple-Cell-Phones-Smartphones/9355/bn_319682'], [59, 'Getting started with iOS', 'https://support.zoom.com/hc/en/article?id=zm_kb&sysparm_article=KB0063582'], [60, 'Use Phone Link to Sync Your Android or iPhone to Your Windows ...', 'https://www.microsoft.com/en-gb/windows/sync-across-your-devices'], [61, 'How to transfer data from an iPhone or iPad to a new Galaxy device ...', 'https://www.samsung.com/uk/support/mobile-devices/how-to-transfer-data-from-an-iphone-or-ipad-to-a-new-galaxy-device-with-smart-switch/'], [62, 'Set up an Outlook account on the iOS Mail app - Microsoft Support', 'https://support.microsoft.com/en-us/office/set-up-an-outlook-account-on-the-ios-mail-app-7e5b180f-bc8f-45cc-8da1-5cefc1e633d1'], [63, "Buy Apple's latest iPhone 16 Pro - CelcomDigi", 'https://www.celcomdigi.com/devices/apple'], [64, 'iPhone - Yes | First to 5G', 'https://www.yes.my/iphone/'], [65, 'iPhone. Meet the next generation. One NZ.', 'https://one.nz/iphone/'], [66, 'Second Hand iPhone Products - iStore Pre-owned iPhone', 'https://istorepreowned.co.za/collections/iphone'], [67, 'Apple iPhone sales worldwide 2007-2023 | Statista', 'https://www.statista.com/statistics/276306/global-apple-iphone-sales-since-fiscal-year-2007/'], [68, 'The iPhone Series | Power Mac Center', 'https://powermaccenter.com/pages/view-all-iphone'], [69, 'Q-Up Tampa - Set iPhone Permissions | City of Tampa', 'https://www.tampa.gov/document/q-tampa-set-iphone-permissions-106276'], [70, 'VPN for iPhone - Protect your data and stay secure - Surfshark', 'https://surfshark.com/blog/what-is-vpn-on-iphone'], [71, 'iPhone Archives - Educational Administration & Human Resource ...', 'https://eahr.tamu.edu/wpa-stats-type/iphone/'], [72, 'Alert! Watch out for a new iPhone scammer tactic. - Information ...', 'https://is.wfu.edu/alert-watch-out-for-a-new-iphone-scammer-tactic/'], [73, 'iPhone (Safari)', 'https://www.lawa.org/-/media/lawa-web/environment/files/noise-mgt/app-instructions-for-iphone-vny-1.ashx'], [74, 'All Apple phones', 'https://www.gsmarena.com/apple-phones-48.php'], [75, 'Article - Connect iPhone or iOS Devic...', 'https://help.sdstate.edu/TDClient/2744/Portal/KB/ArticleDet?ID=134783'], [76, 'Chargers - iPad or iPhone | Hirsh Library', 'https://hirshlibrary.tufts.edu/find-borrow/resources/equipment/chargers-ipad-or-iphone'], [77, 'Refurbished iPhones: choose your model | any model available ...', 'https://swappie.com/ie/iphone/'], [78, 'iPhone no longer works with going from Meeting Roo... - Zoom ...', 'https://community.zoom.com/t5/Zoom-Meetings/iPhone-no-longer-works-with-going-from-Meeting-Room-to-Meeting/td-p/195475'], [79, 'iPhone Users and Sales Stats for 2024', 'https://backlinko.com/iphone-users'], [80, 'How to build an iPhone app | Article | Denison University', 'https://denison.edu/feature/39223'], [81, 'USER GUIDE ADDING A STUDENT', 'https://www.chelseaschools.com/cms/lib/MA50000536/Centricity/Domain/179/WMK%20Account%20Use%20-%20English%20-%20iPhone.pdf'], [82, 'iPhone collection – Machines', 'https://www.machines.com.my/pages/iphone'], [83, 'HubSpot Community - iPhone 14 - Deal Stages dropdown blank ...', 'https://community.hubspot.com/t5/Mobile-Application/iPhone-14-Deal-Stages-dropdown-blank/m-p/972548'], [84, 'How to Take and Edit Awesome iPhone Photos! Technology Module ...', 'https://www.brandeis.edu/bolli/courses-programs/course-descriptions/thursday/art4-5a-thu1.html'], [85, 'Iphone - Amazon.ca', 'https://www.amazon.ca/iphone/s?k=iphone'], [86, 'iPhone 15 | iPhone 14 | iPhone 13 | iPhone SE | Accessories – Maple', 'https://www.maplestore.in/pages/view-all-iphone'], [87, 'iPhone 15 screen protector with blue light filter | Belkin PH', 'https://www.belkin.com/ph/ultraglass-2-blue-light-filter-screen-protector-for-iphone-16-iphone-15-iphone-14-pro/OVA139zz.html'], [88, 'Apple iPhone 13 (128GB) - Midnight : Amazon.in: Electronics', 'https://www.amazon.in/Apple-iPhone-13-128GB-Midnight/dp/B09G9HD6PD'], [89, 'Article - Configuring iPhone/iPad to ...', 'https://bowdoin.teamdynamix.com/TDClient/1814/Portal/KB/ArticleDet?ID=106885'], [90, 'Latest Apple iPhone Deals & Pay Monthly Contracts | Tesco Mobile', 'https://www.tescomobile.com/shop/apple'], [91, 'Browsing by Category iPhone 6 & 6 Plus - GROK Browse', 'https://grok.lsu.edu/browse.aspx?parentCategoryId=3244'], [92, 'Shop For Latest Apple iPhone Mobile Devices | Best Buy Canada', 'https://www.bestbuy.ca/en-ca/category/iphone/36586'], [93, 'New Apple security feature reboots iPhones after 3 days ...', 'https://techcrunch.com/2024/11/14/new-apple-security-feature-reboots-iphones-after-3-days-researchers-confirm/'], [94, "The evolution of Apple's iPhone – Computerworld", 'https://www.computerworld.com/article/1622162/evolution-of-apple-iphone.html'], [95, 'iPhone | Ireland', 'https://www.harveynorman.ie/computing/apple/iphone/'], [96, 'Apple iPhones – Buy the Latest iPhone Models at Officeworks ...', 'https://www.officeworks.com.au/shop/officeworks/c/technology/mobile-phones/apple-iphones'], [97, 'Compra el iPhone 16, 15, 14, 13 y más | iShop Colombia', 'https://co.tiendasishop.com/pages/todos-los-iphone'], [98, 'Admissions iPhone App - UGA Undergraduate Admissions', 'https://www.admissions.uga.edu/blog/admissions-iphone-app/'], [99, 'iPhone', 'https://beyondthebox.ph/collections/iphone'], [100, 'HTC Desire 555 (Cricket) - Transferring iPhone content to your HTC ...', 'https://www.htc.com/us/support/htc-desire-555-cricket/howto/transferring-iphone-content-thru-htc-sync-manager.html']]
Retrieves Google search results for a specified query using the Custom Search API and saves them into an Excel file.¶
import openpyxl
import requests
# Define the search query term
query = 'IPhone 15'
# Create a new Excel workbook
excel_file = openpyxl.Workbook()
# Select the active sheet in the workbook
excel_sheet = excel_file.active
# Loop through 10 pages of search results (Google Custom Search API returns 10 results per page)
for num in range(10):
# Construct the API URL with the required parameters: API key, search engine ID, query, and start index
google_open_api = f'https://www.googleapis.com/customsearch/v1?key={GOOGLE_API_KEY}&cx={GOOGLE_CX}&q={query}&start={num*10+1}'
# Send a GET request to the Google Custom Search API
res = requests.get(google_open_api)
# Check if the response status code indicates success
if res.status_code == 200:
# Parse the JSON response
data = res.json()
# Iterate over the 'items' in the response data to extract search results
for index, item in enumerate(data['items']):
# Append each search result (rank, title, link) to the Excel sheet
excel_sheet.append([num*10+index + 1, item['title'], item['link']])
else:
# Print an error message if the API request fails
print("Error Code:", res.status_code)
# Save the collected search results to an Excel file with a query-based filename
excel_file.save(f"google_search_{query.replace(' ', '_')}.xlsx")
# Close the Excel workbook to release resources
excel_file.close()
[[1, 'iPhone - Apple', 'https://www.apple.com/iphone/'], [2, 'iPhone - Wikipedia', 'https://en.wikipedia.org/wiki/IPhone'], [3, 'Apple', 'https://www.apple.com/'], [4, 'Apple iPhone - Walmart.com', 'https://www.walmart.com/browse/cell-phones/apple-iphone/1105910_7551331_1127173'], [5, 'iPhone - Compare Models - Apple', 'https://www.apple.com/iphone/compare/'], [6, 'Update your iPhone or iPad - Apple Support', 'https://support.apple.com/en-us/118575'], [7, 'Telegram Messenger on the App Store', 'https://apps.apple.com/us/app/telegram-messenger/id686449807'], [8, 'r/iPhone', 'https://www.reddit.com/r/iphone/'], [9, 'iPhone Repair and Service - Apple Support', 'https://support.apple.com/iphone/repair'], [10, 'WhatsApp Messenger on the App Store', 'https://apps.apple.com/us/app/whatsapp-messenger/id310633997'], [11, 'All iPhone - Best Buy', 'https://www.bestbuy.com/site/iphone/all-iphone/pcmcat1683750935583.c?id=pcmcat1683750935583'], [12, 'IPPAWARDS | iPhone Photography Awards – Celebrating the ...', 'https://www.ippawards.com/'], [13, 'Iphone - Amazon.com', 'https://www.amazon.com/iphone/s?k=iphone'], [14, 'New Apple iPhones for Sale: See Pricing & More or Buy Today | T ...', 'https://www.t-mobile.com/cell-phones/brand/apple'], [15, 'Find Devices - Apple iCloud', 'https://www.icloud.com/find/'], [16, '22 New Things Your iPhone Can Do in iOS 18.2 - MacRumors', 'https://www.macrumors.com/2024/12/23/new-things-your-iphone-can-do-in-ios-18-2/'], [17, 'BookBook vol. 2 for iPhone | Leather wallet case with removable shell', 'https://www.twelvesouth.com/products/bookbook-vol-2-for-iphone'], [18, 'SAP Concur Mobile App User Resources - iPhone | SAP Concur ...', 'https://www.concurtraining.com/toolkit/en/mobile/iphone/end-user'], [19, "Check your device's coverage", 'https://checkcoverage.apple.com/'], [20, 'iOS 18 - Apple Developer', 'https://developer.apple.com/ios/'], [21, 'iOS 18 - Apple Developer', 'https://developer.apple.com/ios/'], [22, 'Duo Mobile on iOS - Guide to Two-Factor Authentication · Duo Security', 'https://guide.duo.com/iphone'], [23, 'How to: Encrypt Your iPhone | Surveillance Self-Defense', 'https://ssd.eff.org/module/how-encrypt-your-iphone'], [24, 'Where is "Favorite" in iPhone Photos app … - Apple Community', 'https://discussions.apple.com/thread/255762315'], [25, 'The iOS VoIP App •• iPhone User Manual | 3CX', 'https://www.3cx.com/user-manual/installation-iphone/'], [26, 'Manage your location settings in Chrome - iPhone & iPad - Google ...', 'https://support.google.com/chrome/answer/142065?hl=en&co=GENIE.Platform%3DiOS'], [27, 'FBI Warns iPhone And Android Users—Stop Sending Texts', 'https://www.forbes.com/sites/zakdoffman/2024/12/06/fbi-warns-iphone-and-android-users-stop-sending-texts/'], [28, 'Apple iPhone Repair Help: Learn How to Fix It Yourself.', 'https://www.ifixit.com/Device/iPhone'], [29, 'MFi Program', 'https://mfi.apple.com/'], [30, 'iPhone | The Guardian', 'https://www.theguardian.com/technology/iphone'], [31, 'iPhone Photography School | iPhone Photography Online Courses', 'https://iphonephotographyschool.com/'], [32, 'Introducing iPhone 16 | Apple - YouTube', 'https://www.youtube.com/watch?v=GDlkCkcIqTs'], [33, 'Apple iPhone | endoflife.date', 'https://endoflife.date/iphone'], [34, 'Best iPhone in 2024: Which Apple Phone Should You Buy? - CNET', 'https://www.cnet.com/tech/mobile/best-iphone/'], [35, 'Download Epic Games for iOS & iPhone - Epic Games Store', 'https://store.epicgames.com/en-US/mobile/ios'], [36, 'Apple iPhone: Shop Apple Smartphones | Verizon', 'https://www.verizon.com/smartphones/apple/'], [37, 'How to transfer data from an iPhone or iPad to a new Galaxy device ...', 'https://www.samsung.com/uk/support/mobile-devices/how-to-transfer-data-from-an-iphone-or-ipad-to-a-new-galaxy-device-with-smart-switch/'], [38, 'iPhone Cases – CASETiFY', 'https://www.casetify.com/iphone-cases'], [39, 'Choose an iPhone / IPSW Downloads', 'https://ipsw.me/product/iPhone'], [40, 'How to check read receipts | WhatsApp Help Center', 'https://faq.whatsapp.com/665923838265756'], [41, 'Certified Refurbished iPhone | Back Market', 'https://www.backmarket.com/en-us/l/iphone/e8724fea-197e-4815-85ce-21b8068020cc'], [42, 'Accept payments with Tap to Pay on iPhone | Square Support ...', 'https://squareup.com/help/us/en/article/7786-get-started-with-tap-to-pay-on-iphone'], [43, 'iPhone Skins » dbrand', 'https://dbrand.com/shop/devices/iphone-skins'], [44, 'Download the Gemini app for iPhone today', 'https://blog.google/products/gemini/gemini-iphone-app/'], [45, "MacRumors Buyer's Guide: Know When to Buy iPhone, Mac, iPad", 'https://buyersguide.macrumors.com/'], [46, 'Best iPhones 2024: Which iPhone model should you buy? | ZDNET', 'https://www.zdnet.com/article/best-iphone/'], [47, "What's the Best iPhone to Buy or Avoid Right Now? (2024) | WIRED", 'https://www.wired.com/gallery/iphone-buying-guide/'], [48, 'New Apple iPhones, including iPhone 16: Get Prices & Buy Now ...', 'https://www.att.com/buy/phones/browse/apple/'], [49, 'Clicks Keyboard for iPhone', 'https://www.clicks.tech/'], [50, 'iPhone - 2nd Hand Devices | CompAsia Malaysia', 'https://compasia.my/collections/iphone'], [51, 'Install and set up Office on an iPhone or iPad - Microsoft Support', 'https://support.microsoft.com/en-us/office/install-and-set-up-office-on-an-iphone-or-ipad-9df6d10c-7281-4671-8666-6ca8e339b628'], [52, 'The Ultimate Guide To iPhone Resolutions', 'https://www.paintcodeapp.com/news/ultimate-guide-to-iphone-resolutions'], [53, "How China Built 'iPhone City' With Billions in Perks for Apple's ...", 'https://www.nytimes.com/2016/12/29/technology/apple-iphone-china-foxconn.html'], [54, 'Apple iPhone 12 Prepaid | Price, Specs & Deals | Metro by T-Moblie', 'https://www.metrobyt-mobile.com/cell-phone/apple-iphone-12'], [55, 'Getting started with iOS', 'https://support.zoom.com/hc/en/article?id=zm_kb&sysparm_article=KB0063582'], [56, 'iPhones for Sale - New & Used iPhones at Great Prices - eBay', 'https://www.ebay.com/b/Apple-Cell-Phones-Smartphones/9355/bn_319682'], [57, 'How to Play Fortnite on iPhone iOS or iPad', 'http://www.fortnite.com/mobile/ios?lang=en-US'], [58, 'Use Phone Link to Sync Your Android or iPhone to Your Windows ...', 'https://www.microsoft.com/en-gb/windows/sync-across-your-devices'], [59, "Buy Apple's latest iPhone 16 Pro - CelcomDigi", 'https://www.celcomdigi.com/devices/apple'], [60, 'iPhone - Yes | First to 5G', 'https://www.yes.my/iphone/'], [61, 'Second Hand iPhone Products - iStore Pre-owned iPhone', 'https://istorepreowned.co.za/collections/iphone'], [62, 'Apple iPhone sales worldwide 2007-2023 | Statista', 'https://www.statista.com/statistics/276306/global-apple-iphone-sales-since-fiscal-year-2007/'], [63, 'The iPhone Series | Power Mac Center', 'https://powermaccenter.com/pages/view-all-iphone'], [64, 'Q-Up Tampa - Set iPhone Permissions | City of Tampa', 'https://www.tampa.gov/document/q-tampa-set-iphone-permissions-106276'], [65, 'VPN for iPhone - Protect your data and stay secure - Surfshark', 'https://surfshark.com/blog/what-is-vpn-on-iphone'], [66, 'iPhone Archives - Educational Administration & Human Resource ...', 'https://eahr.tamu.edu/wpa-stats-type/iphone/'], [67, 'Alert! Watch out for a new iPhone scammer tactic. - Information ...', 'https://is.wfu.edu/alert-watch-out-for-a-new-iphone-scammer-tactic/'], [68, 'iPhone (Safari)', 'https://www.lawa.org/-/media/lawa-web/environment/files/noise-mgt/app-instructions-for-iphone-vny-1.ashx'], [69, 'All Apple phones', 'https://www.gsmarena.com/apple-phones-48.php'], [70, '"The iPhone Meets the Fourth Amendment" by Adam M. Gershowitz', 'https://scholarship.law.wm.edu/facpubs/1253/'], [71, 'Article - Connect iPhone or iOS Devic...', 'https://help.sdstate.edu/TDClient/2744/Portal/KB/ArticleDet?ID=134783'], [72, 'iPhone. Meet the next generation. One NZ.', 'https://one.nz/iphone/'], [73, 'Chargers - iPad or iPhone | Hirsh Library', 'https://hirshlibrary.tufts.edu/find-borrow/resources/equipment/chargers-ipad-or-iphone'], [74, 'iPhone no longer works with going from Meeting Roo... - Zoom ...', 'https://community.zoom.com/t5/Zoom-Meetings/iPhone-no-longer-works-with-going-from-Meeting-Room-to-Meeting/td-p/195475'], [75, 'iPhone Users and Sales Stats for 2024', 'https://backlinko.com/iphone-users'], [76, 'How to build an iPhone app | Article | Denison University', 'https://denison.edu/feature/39223'], [77, 'USER GUIDE ADDING A STUDENT', 'https://www.chelseaschools.com/cms/lib/MA50000536/Centricity/Domain/179/WMK%20Account%20Use%20-%20English%20-%20iPhone.pdf'], [78, 'iPhone collection – Machines', 'https://www.machines.com.my/pages/iphone'], [79, 'Refurbished iPhones: choose your model | any model available ...', 'https://swappie.com/ie/iphone/'], [80, 'HubSpot Community - iPhone 14 - Deal Stages dropdown blank ...', 'https://community.hubspot.com/t5/Mobile-Application/iPhone-14-Deal-Stages-dropdown-blank/m-p/972548'], [81, 'How to Take and Edit Awesome iPhone Photos! Technology Module ...', 'https://www.brandeis.edu/bolli/courses-programs/course-descriptions/thursday/art4-5a-thu1.html'], [82, 'iPhone 15 | iPhone 14 | iPhone 13 | iPhone SE | Accessories – Maple', 'https://www.maplestore.in/pages/view-all-iphone'], [83, 'iPhone 15 screen protector with blue light filter | Belkin PH', 'https://www.belkin.com/ph/ultraglass-2-blue-light-filter-screen-protector-for-iphone-16-iphone-15-iphone-14-pro/OVA139zz.html'], [84, 'Latest iPhone Pay Monthly Deals & Contracts | EE Shop', 'https://ee.co.uk/mobile/pay-monthly-phones-gallery/apple-iphone'], [85, 'Iphone - Amazon.ca', 'https://www.amazon.ca/iphone/s?k=iphone'], [86, 'How to Transfer iPhone Contacts to Android | Android', 'https://www.android.com/articles/transfer-contacts/'], [87, 'Article - Configuring iPhone/iPad to ...', 'https://bowdoin.teamdynamix.com/TDClient/1814/Portal/KB/ArticleDet?ID=106885'], [88, 'Apple iPhone 13 (128GB) - Midnight : Amazon.in: Electronics', 'https://www.amazon.in/Apple-iPhone-13-128GB-Midnight/dp/B09G9HD6PD'], [89, 'Browsing by Category iPhone 6 & 6 Plus - GROK Browse', 'https://grok.lsu.edu/browse.aspx?parentCategoryId=3244'], [90, 'Latest Apple iPhone Deals & Pay Monthly Contracts | Tesco Mobile', 'https://www.tescomobile.com/shop/apple'], [91, 'Apple iPhones – Buy the Latest iPhone Models at Officeworks ...', 'https://www.officeworks.com.au/shop/officeworks/c/technology/mobile-phones/apple-iphones'], [92, 'New Apple security feature reboots iPhones after 3 days ...', 'https://techcrunch.com/2024/11/14/new-apple-security-feature-reboots-iphones-after-3-days-researchers-confirm/'], [93, 'iPhones For Sale Online in NZ - Noel Leeming', 'https://www.noelleeming.co.nz/c/mobile-smart-devices/phones/smartphones/iphone'], [94, "The evolution of Apple's iPhone – Computerworld", 'https://www.computerworld.com/article/1622162/evolution-of-apple-iphone.html'], [95, 'iPhone Health Records Now Available for U.K. TrakCare Users ...', 'https://www.intersystems.com/resources/iphone-health-records-now-available-for-uk-trakcare-users/'], [96, 'Device Support | Apple iPhone 11 | Xfinity Mobile', 'https://www.xfinity.com/mobile/support/device-troubleshooting?page=device/apple/iphone-11-ios-18'], [97, 'Admissions iPhone App - UGA Undergraduate Admissions', 'https://www.admissions.uga.edu/blog/admissions-iphone-app/'], [98, 'Compra el iPhone 16, 15, 14, 13 y más | iShop Colombia', 'https://co.tiendasishop.com/pages/todos-los-iphone'], [99, 'Shop Apple iPhones - Find iPhone 16, 15, 14 Series & More - JB Hi ...', 'https://www.jbhifi.co.nz/collections/mobile-phones/iphones'], [100, 'Shop Apple iPhone Range | Free Same Day Delivery', 'https://www.istore.co.za/iphone/shop-iphone-range']]
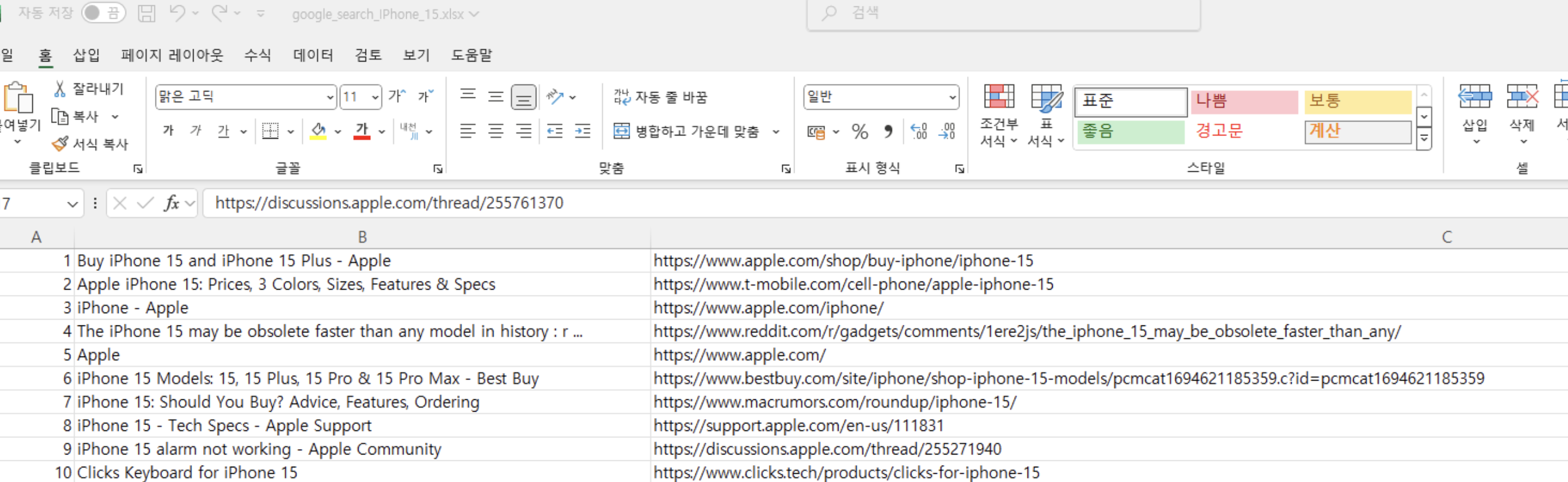